What is MVC?
MVC is a framework pattern that splits an application's implementation logic into three component roles: models, views, and controllers.
Explain MVC Architecture?
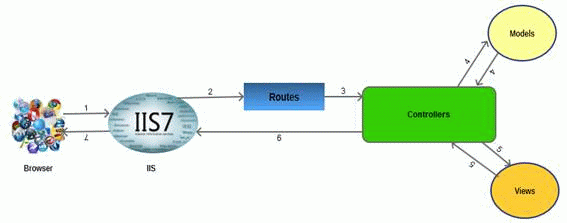
The architecture is self-explanatory. The browser (as usual) sends a request to IIS, IIS searches for the route defined in the MVC application and passes the request to the controller as specified by the route, the controller communicates with the model and passes the populated model (entity) to View (front end), Views are populated with model properties, and are rendered on the browser, passing the response to the browser through IIS via controllers that invoked the specified View.
What are the new features of MVC 2?
ASP.NET MVC 2 was released in March 2010. Its main features are:
ASP.NET MVC 3 shipped just 10 months after MVC 2 in Jan 2011. Some of the top features in MVC 3 included:
The following are the top features of MVC 4:
The following processes are performed by ASP.NET MVC page::
As per Wikipedia 'the process of breaking a computer program into distinct features that overlap in functionality as little as possible'. The MVC design pattern aims to separate content from presentation and data-processing from content.
Where do we see Separation of Concerns in MVC?
Between the data-processing (Model) and the rest of the application.
When we talk about Views and Controllers, their ownership itself explains separate. The views are just the presentation form of an application, it does not need to know specifically about the requests coming from the controller. The Model is independent of View and Controllers, it only holds business entities that can be passed to any View by the controller as required for exposing them to the end user. The controller is independent of Views and Models, its sole purpose is to handle requests and pass it on as per the routes defined and as per the need of rendering the views. Thus our business entities (model), business logic (controllers) and presentation logic (views) lie in logical/physical layers independent of each other.
What is Razor View Engine?
Razor is the first major update to render HTML in MVC 3. Razor was designed specifically as a view engine syntax. It has one main focus: code-focused templating for HTML generation. Here's how that same markup would be generated using Razor:@model MvcMusicStore.Models.Genre@{ViewBag.Title = "Browse Albums";}<div class="genre"><h3><em>@Model.Name</em> Albums</h3><ul id="album-list">@foreach (var album in Model.Albums)
{<li>
<a href="@Url.Action("Details", new { id = album.AlbumId })"><img alt="@album.Title" src="@album.AlbumArtUrl" /><span>@album.Title</span></a>
</li>}</ul>
</div>
The Razor syntax is easier to type, and easier to read. Razor doesn't have the XML-like heavy syntax of the Web Forms view engine.
What is Unobtrusive JavaScript?
Unobtrusive JavaScript is a general term that conveys a general philosophy, similar to the term REST (Representational State Transfer). The high-level description is that unobtrusive JavaScript doesn't intermix JavaScript code in your page markup. For example, rather than hooking in via event attributes like onclick and onsubmit, the unobtrusive JavaScript attaches to elements by their ID or class, often based on the presence of other attributes (such as HTML5 data-attributes).
It's got semantic meaning and all of it; the tag structure, element attributes and so on should have a precise meaning. Strewing JavaScript gunk across the page to facilitate interaction (I'm looking at you, -doPostBack!) harms the content of the document.
What is JSON Binding?
MVC 3 included JavaScript Object Notation (JSON) binding support via the new JsonValueProviderFactory, enabling the action methods to accept and model-bind data in JSON format. This is especially useful in advanced Ajax scenarios like client templates and data binding that need to post data back to the server.
What is Dependency Resolution?
MVC 3 introduced a new concept called a dependency resolver, that greatly simplified the use of dependency injection in your applications. This made it easier to decouple application components, making them more configurable and easier to test.
Support was added for the following scenarios:
Display modes use a convention-based approach to allow selecting various views based on the browser making the request. The default view engine first looks for views with names ending with ".Mobile.cshtml" when the browser's user agent indicates a known mobile device. For example, if we have a generic view titled "Index.cshtml" and a mobile view titled "Index.Mobile.cshtml" then MVC 4 will automatically use the mobile view when viewed in a mobile browser.
Additionally, we can register your own custom device modes that will be based on your own custom criteria, all in just one code statement. For example, to register a WinPhone device mode that would serve views ending with ".WinPhone.cshtml" to Windows Phone devices, you'd use the following code in the Application_Start method of your Global.asax:DisplayModeProvider.Instance.Modes.Insert(0, new DefaultDisplayMode("WinPhone")
{ContextCondition = (context => context.GetOverriddenUserAgent().IndexOf
("Windows Phone OS", StringComparison.OrdinalIgnoreCase) >= 0)});
What is AuthConfig.cs in MVC 4?
"AuthConfig.cs" configures security settings, including sites for OAuth login.
What is BundleConfig.cs in MVC 4?
"BundleConfig.cs" in MVC4 registers bundles used by the bundling and minification system. Several bundles are added by default, including jQuery, jQueryUI, jQuery validation, Modernizr, and default CSS references.
What is FilterConfig.cs in MVC 4?
This is used to register global MVC filters. The only filter registered by default is the HandleErrorAttribute, but this is a great place to put other filter registrations.
What is RouteConfig.cs in MVC 4?
"RouteConfig.cs" holds the granddaddy of the MVC config statements and Route configuration.
What is WebApiConfig.cs in MVC 4?
Used to register Web API routes, as well as set any additional Web API configuration settings.
What's new for adding a controller in a MVC 4 application?
Previously (in MVC 3 and MVC 2), the Visual Studio Add Controller menu item only displayed when we right-clicked on the Controllers folder. However, the use of the Controllers folder was purely for organization. (MVC will recognize any class that implements the IController interface as a Controller, regardless of its location in your application.) The MVC 4 Visual Studio tooling has been modified to display the Add Controller menu item for any folder in your MVC project. This allows us to organize controllers however you would like, perhaps separating them into logical groups or separating MVC and Web API controllers.
What are the software requirements of an ASP.NET MVC 4 application?
MVC 4 runs on the following Windows client operating systems:
What are the various types of Application Templates used to create an MVC application?
The various templates are as follows:
The default Top-level directories are:
ASP.NET MVC namespaces as well as classes are located in assembly System.Web.Mvc.
Note: Some of the content has been taken from various books/articles.
What is System.Web.Mvc namespace?
This namespace contains classes and interfaces that support the MVC pattern for ASP.NET Web applications. This namespace includes classes that represent controllers, controller
factories, action results, views, partial views, and model binders.
What is System.Web.Mvc.Ajax namespace?
The System.Web.Mvc.Ajax namespace contains classes that support Ajax scripting in an ASP.NET MVC application. The namespace includes support for Ajax scripts and Ajax option settings as well.
What is System.Web.Mvc.Async namespace?
The System.Web.Mvc.Async namespace contains classes and interfaces that support asynchronous actions in an ASP.NET MVC application.
What is System.Web.Mvc.Html namespace?
The System.Web.Mvc.Html namespace contains classes that help render HTML controls in an MVC application. This namespace includes classes that support forms, input controls, links, partial views, and validation.
What is ViewData, ViewBag and TempData?
MVC provides ViewData, ViewBag and TempData for passing data from the controller, view and in subsequent requests as well. ViewData and ViewBag are similar to some extent but TempData performs additional roles.
What are the roles and similarities between ViewData and ViewBag?
One obvious difference is that ViewBag works only when the key being accessed is a valid C# identifier. For example, if you place a value in ViewData["KeyWith Spaces"] then you can't access that value using ViewBag because the code won't compile.
Another key issue to be aware of is that dynamic values cannot be passed in as parameters to extension methods. The C# compiler must know the real type of every parameter at compile time in order for it to choose the correct extension method.
If any parameter is dynamic then compilation will fail. For example, this code will always fail: @Html.TextBox("name", ViewBag.Name). To work around this, either use ViewData["Name"] or cast the value to a specifi c type: (string) ViewBag.Name.
What is TempData?
TempData is a dictionary derived from the TempDataDictionary class and stored in a short-lived session. It is a string key and object value.
It maintains the information for the duration of an HTTP Request. This means only from one page to another. It helps to maintain data when we move from one controller to another controller or from one action to another action. In other words, when we redirect Tempdata, it helps to maintain the data between those redirects. It internally uses session variables. Temp data used during the current and subsequent request only means it is used when we are sure that the next request will be redirecting to the next view. It requires typecasting for complex data types and checks for null values to avoid errors. Generally it is used to store only one-time messages, like error messages and validation messages.
How can you define a dynamic property using viewbag in ASP.NET MVC?
Assign a key name with the syntax "ViewBag.[Key]=[ Value]" and value using the equal to operator.
For example, you need to assign a list of students to the dynamic Students property of ViewBag as in the following:List<string> students = new List<string>();countries.Add("Akhil");countries.Add("Ekta");
ViewBag.Students = students;//Students is a dynamic property associated with ViewBag.
Note: Some of the content has been taken from various books/articles.
What is ViewModel (taken from stackoverflow)?
Accepted A view model represents data that you want to have displayed on your view/page.
Let's say that you have an Employee class that represents your employee domain model and it contains the following 4 properties:public class Employee : IEntity
{
public int Id { get; set; } // Employee's unique identifier public string FirstName { get; set; } // Employee's first name public string LastName { get; set; } // Employee's last name public DateTime DateCreated { get; set; } // Date when employee was created}
View models differ from domain models in that view models only contain the data (represented by properties) that you want to use on your view. For example, let's say that you want to add a new employee record, your view model might look like this:public class CreateEmployeeViewModel{
public string FirstName { get; set; }
public string LastName { get; set; }}
As you can see it only contains 2 of the properties of the employee domain model. Why is this you may ask? Id might not be set from the view and it might be auto-generated by the Employee table. And DateCreated might also be set in the Stored Procedure or in the service layer of your application. So Id and DateCreated is not needed in the view model.
When loading the view/page, the create action method in your employee controller will create an instance of this view model, populate any fields if required, and then pass this view model to the view:public class EmployeeController : Controller
{
private readonly IEmployeeService employeeService;
public EmployeeController(IEmployeeService employeeService)
{
this.employeeService = employeeService;
}
public ActionResult Create()
{
CreateEmployeeViewModel viewModel = new CreateEmployeeViewModel();
return View(viewModel);
}
public ActionResult Create(CreateEmployeeViewModel viewModel)
{
// Do what ever needs to be done before adding the employee to the database }}
Your view might look like this (assuming you are using ASP.NET MVC3 and razor):@model MyProject.Web.ViewModels.ProductCreateViewModel <table>
<tr>
<td><b>First Name:</b></td>
<td>@Html.TextBoxFor(x => x.FirstName, new { maxlength = "50", size = "50" })@Html.ValidationMessageFor(x => x.FirstName)</td>
</tr>
<tr>
<td><b>Last Name:</b></td>
<td>@Html.TextBoxFor(x => x.LastName, new { maxlength = "50", size = "50" })@Html.ValidationMessageFor(x => x.LastName)</td>
</tr></table>
Validation would thus be done only on FirstName and LastName. Using Fluent Validation you might have validation like this:
public class CreateEmployeeViewModelValidator : AbstractValidator<CreateEmployeeViewModel>
{
public CreateEmployeeViewModelValidator()
{
RuleFor(x => x.FirstName)
.NotEmpty()
.WithMessage("First name required")
.Length(1, 50)
.WithMessage("First name must not be greater than 50 characters");
RuleFor(x => x.LastName)
.NotEmpty()
.WithMessage("Last name required")
.Length(1, 50)
.WithMessage("Last name must not be greater than 50 characters");
}}
The key thing to remember is that the view model only represents the data that you want to use. You can imagine all the unnecessary code and validation if you have a domain model with 30 properties and you only want to update a single value. Given this scenario you would only have this one value/property in the view model and not the entire domain object.
How do you check for AJAX requests with C# in MVC.NET?
The solution is independed of the MVC.NET framework and is global across server side technologies. Most modern AJAX applications utilize XmlHTTPRequest to send async requests to the server. Such requests will have a distinct request header:
X-Requested-With = XMLHTTPREQUEST
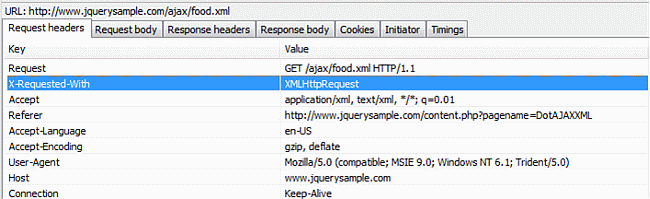
MVC.NET provides helper functions to check for ajax requests that internally inspects the "X-Requested-With" request header to set the "IsAjax" flag.
What are Scaffold templates?
These templates use the Visual Studio T4 templating system to generate a view based on the model type selected. Scaffolding in ASP.NET MVC can generate the boilerplate code we need to create, read, update, and delete (CRUD) functionality in an application. The scaffolding templates can examine the type definition for it then generate a controller and the controller's associated views. The scaffolding knows how to name controllers, how to name views, what code needs to go to each component, and where to place all these pieces in the project for the application to work.
What are the types of Scaffolding Templates?
Show an example of the difference in syntax in Razor and WebForm Views
What is a View Engine?
Ans:- View Engines are responsible for rendering the HTML from your views to the browser. The view engine template will have different syntax for implementation.
What is Razor view engine?
Ans:- The Razor view engine is an advanced view engine from Microsoft, It is launched with MVC 3 (in VS 4.0). Razor using an @ character instead classic ASP.NET(.aspx) <% %>
and Razor does not require you to explicitly close the code-block, this view engine is parsed intelligently by the run-time to determine what is a presentation element and what is a code element.
What are the two popular asp.net mvc view engines?
Ans:- 1. Razor
2. .aspx
What are the file extension for Razore view engine files?
Ans:- Razor syntax have the special file extension cshtml (Razor with C#) and vbhtml (Razor with VB).
Can you give a simple example of textbox?
Ans:- @Html.TextBox("Name")
What HTML code will be produce by "@Html.TextBox("Name")"?
Ans:- It will produce:-
<input id="Name" name="Name" type="textbox" />
What is the difference between @Html.TextBox and @Html.TextBoxFor
Ans:- Finaly both produce the same HTML but Html.TextBoxFor() is strongly typed with any model, where as Html.TextBox isn't.
TextBoxFor input extension is first time introduce in which MVC version?
Ans:- In MVC2
How to add Namespaces in Razor view engine?
Ans:- @using YourCustomNamespace
LINQ
Linq stands for Language Integrated Query
A query is an expression that retrieves data from a data source. Queries are usually expressed in a specialized query language.
Microsoft language developers provided a way to express queries directly in their languages (such as c# and vb).
A query is an expression that retrieves data from a data source. Queries are usually expressed in a specialized query language.
Three Parts of a Query Operation
What are the advantages of hosting WCF Services in IIS as
compared to self-hosting?
There are two main advantages of using IIS over self-hosting:-
Automatic activation
IIS provides automatic activation that means the service is not necessary to be running in
advance. When any message is received by the service it then launches and fulfills the request.
But in case of self hosting the service should always be running.
Process recycling
If IIS finds that a service is not healthy that means if it has memory leaks etc, IIS recycles the
process.
For every browser instance, a worker process is spawned and the request is serviced. When the browser disconnects the worker,
process stops and you loose all information. IIS also restarts the worker process. By default, the
worker process is recycled at around 120 minutes. So why does IIS recycle. By restarting the
worker process it ensures any bad code or memory leak do not cause issue to the whole system.
In case of self-hosting both the above features, you will need to code yourself. Lot of work
right!!.
what are the various ways of hosting a WCF service?
There are three major ways to host a WCF service:-
• Self-hosting the service in his own application domain. This we have already covered in
the first section. The service comes in to existence when you create the object of Service
Host class and the service closes when you call the Close of the Service Host class.
• Host in application domain or process provided by IIS Server.
• Host in Application domain and process provided by WAS (Windows Activation
Service) Server.
What is WCF?
WCF stands for Windows Communication Foundation. It is a Software development kit for developing services on Windows. WCF is introduced in .NET 3.0. in the System.ServiceModel namespace. WCF is based on basic concepts of Service oriented architecture (SOA)
It consists of three main points: Address,Binding and Contract.
Windows Communication Foundation (WCF) is an SDK for developing and deploying services on Windows. WCF provides a runtime environment for services, enabling you to expose CLR types as services, and to consume other services as CLR types.
WCF is part of .NET 3.0 and requires .NET 2.0, so it can only run on systems that support it
WCF(Indigo was the code name for WCF) is a unification of .Net framework communication technologies.
Endpoint
Every service must have Address that defines where the service resides, Contract that defines what the service does and a Binding that defines how to communicate with the service. In WCF the relationship between Address, Contract and Binding is called Endpoint.
The Endpoint is the fusion of Address, Contract and Binding.
Explain Address,Binding and contract for a WCF Service?
Address: Address defines where the service resides.
Binding: Binding defines how to communicate with the service.
Contract: Contract defines what is done by the service.
Address
Address is a way of letting client know that where a service is located. In WCF, every service is associated with a unique address. This contains the location of the service and transport schemas.
WCF supports following transport schemas
HTTP
TCP
Peer network
IPC (Inter-Process Communication over named pipes)
MSMQ
The sample address for above transport schema may look like
http://localhost:81
http://localhost:81/MyService
net.tcp://localhost:82/MyService
net.pipe://localhost/MyPipeService
net.msmq://localhost/private/MyMsMqService
net.msmq://localhost/MyMsMqService
Bindings
A binding defines how an endpoint communicates to the world. A binding defines the transport (such as HTTP or TCP) and the encoding being used (such as text or binary). A binding can contain binding elements that specify details like the security mechanisms used to secure messages, or the message pattern used by an endpoint.
A binding defines how an endpoint communicates to the world. A binding defines the transport (such as HTTP or TCP) and the encoding being used (such as text or binary). A binding can contain binding elements that specify details like the security mechanisms used to secure messages, or the message pattern used by an endpoint.
WCF supports nine types of bindings.
Peer network binding
Offered by the NetPeerTcpBinding class, this uses peer networking as a transport. The peer network-enabled client and services all subscribe to the same grid and broadcast messages to it.
Contracts
In WCF, all services expose contracts. The contract is a platform-neutral and standard way of describing what the service does.
WCF defines four types of contracts.
Service contracts
Describe which operations the client can perform on the service.
There are two types of Service Contracts.
ServiceContract - This attribute is used to define the Interface.
OperationContract
This attribute is used to define the method inside Interface.
Data contracts
Define which data types are passed to and from the service. WCF defines implicit contracts for built-in types such as int and string, but we can easily define explicit opt-in data contracts for custom types.
There are two types of Data Contracts.
DataContract - attribute used to define the class
DataMember - attribute used to define the properties.
If DataMember attributes are not specified for a properties in the class, that property can't be passed to-from web service.
Fault contracts
Define which errors are raised by the service, and how the service handles and propagates errors to its clients.
Message contracts
Allow the service to interact directly with messages. Message contracts can be typed or untyped, and are useful in interoperability cases and when there is an existing message format we have to comply with.
1. IIS
2. Self Hosting
3. WAS (Windows Activation Service)
The below code shows how to expose a RESTful service
Web services can only be invoked by HTTP. While Services or a WCF component can be invoked by any protocol and any transport type. Second web services are not flexible. However Services are flexible. If you make a new version of the service then you need to just expose a new end. Therefore services are agile and which is a very practical approach looking at the current business trends.
Can we have two way communications in MSMQ?
Yes
SOA is a collection of well defined services, where each individual service can be modified independently of other services to help respond to the ever evolving market conditions of a business.
What is the difference between transport level and message level security?
What are the advantages of WCF?
WCF Services client configuration file contains endpoint, address, binding and contract.
What are the features of WCF?
WCF EXAMPLE-1
WCF EXAMPLE-2
WCF EXAMPLE-3
MVC
MVC is one of three ASP.NET programming models.
MVC is a framework for building web applications using a MVC (Model View Controller) design:
The Model-View-Controller (MVC) pattern separates the modeling of the domain, the presentation, and the actions based on user input into three separate classes [Burbeck92]:
Model. The model manages the behavior and data
of the application domain, responds to requests for information about
its state (usually from the view), and responds to instructions to
change state (usually from the controller).
View. The view manages the display of information.
Controller. The controller interprets the mouse
and keyboard inputs from the user, informing the model and/or the view
to change as appropriate.
Model–view–controller (MVC) is a software architecture pattern which separates the representation of information from the user's interaction with it.The model consists of application data, business rules, logic, and functions. A view can be any output representation of data, such as a chart or a diagram. Multiple views of the same data are possible, such as a bar chart for management and a tabular view for accountants. The controller mediates input, converting it to commands for the model or view. The central ideas behind MVC are code reusability and separation of concerns
MVC is a framework pattern that splits an application's implementation logic into three component roles: models, views, and controllers.
- Model: The business entity on which the overall application operates. Many applications use a persistent storage mechanism (such as a database) to store data. MVC does not specifically mention the data access layer because it is understood to be encapsulated by the Model.
- View: The user interface that renders the Model into a form of interaction.
- Controller: Handles a request from a View and updates the Model that results in a change of the Model's state.
Explain MVC Architecture?
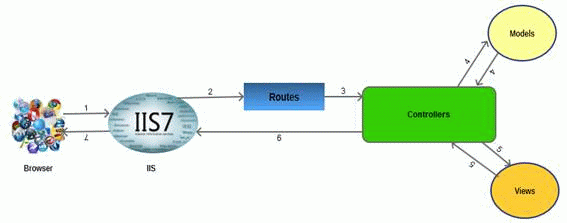
The architecture is self-explanatory. The browser (as usual) sends a request to IIS, IIS searches for the route defined in the MVC application and passes the request to the controller as specified by the route, the controller communicates with the model and passes the populated model (entity) to View (front end), Views are populated with model properties, and are rendered on the browser, passing the response to the browser through IIS via controllers that invoked the specified View.
What are the new features of MVC 2?
ASP.NET MVC 2 was released in March 2010. Its main features are:
- Introduction of UI helpers with automatic scaffolding with customizable templates.
- Attribute-based model validation on both client and server.
- Strongly typed HTML helpers.
- Improved Visual Studio tooling.
- There
were also many API enhancements and "pro" features, based on feedback
from developers building a variety of applications on ASP.NET MVC 1,
such as:
- Support for partitioning large applications into areas.
- Asynchronous controllers support.
- Support for rendering subsections of a page/site using Html.RenderAction.
- Many new helper functions, utilities, and API enhancements.
ASP.NET MVC 3 shipped just 10 months after MVC 2 in Jan 2011. Some of the top features in MVC 3 included:
- The Razor view engine.
- Support for .NET 4 Data Annotations.
- Improved model validation
- Greater control and flexibility with support for dependency resolution and global action filters.
- Better JavaScript support with unobtrusive JavaScript, jQuery Validation, and JSON binding.
- Use of NuGet to deliver software and manage dependencies throughout the platform.
The following are the top features of MVC 4:
- ASP.NET Web API.
- Enhancements to default project templates.
- Mobile project template using jQuery Mobile.
- Display Modes.
- Task support for Asynchronous Controllers.
- Bundling and minification.
The following processes are performed by ASP.NET MVC page::
- App initialization
- Routing
- Instantiate and execute controller
- Locate and invoke controller action
- Instantiate and render view
- Provides a clean separation of concerns among UI (Presentation layer), model (Transfer objects/Domain Objects/Entities) and Business Logic (Controller).
- Easy to UNIT Test.
- Improved reusability of views/model. One can have multiple views that can point to the same model and vice versa.
- Improved structuring of the code.
As per Wikipedia 'the process of breaking a computer program into distinct features that overlap in functionality as little as possible'. The MVC design pattern aims to separate content from presentation and data-processing from content.
Where do we see Separation of Concerns in MVC?
Between the data-processing (Model) and the rest of the application.
When we talk about Views and Controllers, their ownership itself explains separate. The views are just the presentation form of an application, it does not need to know specifically about the requests coming from the controller. The Model is independent of View and Controllers, it only holds business entities that can be passed to any View by the controller as required for exposing them to the end user. The controller is independent of Views and Models, its sole purpose is to handle requests and pass it on as per the routes defined and as per the need of rendering the views. Thus our business entities (model), business logic (controllers) and presentation logic (views) lie in logical/physical layers independent of each other.
What is Razor View Engine?
Razor is the first major update to render HTML in MVC 3. Razor was designed specifically as a view engine syntax. It has one main focus: code-focused templating for HTML generation. Here's how that same markup would be generated using Razor:@model MvcMusicStore.Models.Genre@{ViewBag.Title = "Browse Albums";}<div class="genre"><h3><em>@Model.Name</em> Albums</h3><ul id="album-list">@foreach (var album in Model.Albums)
{<li>
<a href="@Url.Action("Details", new { id = album.AlbumId })"><img alt="@album.Title" src="@album.AlbumArtUrl" /><span>@album.Title</span></a>
</li>}</ul>
</div>
The Razor syntax is easier to type, and easier to read. Razor doesn't have the XML-like heavy syntax of the Web Forms view engine.
What is Unobtrusive JavaScript?
Unobtrusive JavaScript is a general term that conveys a general philosophy, similar to the term REST (Representational State Transfer). The high-level description is that unobtrusive JavaScript doesn't intermix JavaScript code in your page markup. For example, rather than hooking in via event attributes like onclick and onsubmit, the unobtrusive JavaScript attaches to elements by their ID or class, often based on the presence of other attributes (such as HTML5 data-attributes).
It's got semantic meaning and all of it; the tag structure, element attributes and so on should have a precise meaning. Strewing JavaScript gunk across the page to facilitate interaction (I'm looking at you, -doPostBack!) harms the content of the document.
What is JSON Binding?
MVC 3 included JavaScript Object Notation (JSON) binding support via the new JsonValueProviderFactory, enabling the action methods to accept and model-bind data in JSON format. This is especially useful in advanced Ajax scenarios like client templates and data binding that need to post data back to the server.
What is Dependency Resolution?
MVC 3 introduced a new concept called a dependency resolver, that greatly simplified the use of dependency injection in your applications. This made it easier to decouple application components, making them more configurable and easier to test.
Support was added for the following scenarios:
- Controllers (registering and injecting controller factories, injecting controllers)
- Views (registering and injecting view engines, injecting dependencies into view pages)
- Action filters (locating and injecting filters)
- Model binders (registering and injecting)
- Model validation providers (registering and injecting)
- Model metadata providers (registering and injecting)
- Value providers (registering and injecting)
Display modes use a convention-based approach to allow selecting various views based on the browser making the request. The default view engine first looks for views with names ending with ".Mobile.cshtml" when the browser's user agent indicates a known mobile device. For example, if we have a generic view titled "Index.cshtml" and a mobile view titled "Index.Mobile.cshtml" then MVC 4 will automatically use the mobile view when viewed in a mobile browser.
Additionally, we can register your own custom device modes that will be based on your own custom criteria, all in just one code statement. For example, to register a WinPhone device mode that would serve views ending with ".WinPhone.cshtml" to Windows Phone devices, you'd use the following code in the Application_Start method of your Global.asax:DisplayModeProvider.Instance.Modes.Insert(0, new DefaultDisplayMode("WinPhone")
{ContextCondition = (context => context.GetOverriddenUserAgent().IndexOf
("Windows Phone OS", StringComparison.OrdinalIgnoreCase) >= 0)});
What is AuthConfig.cs in MVC 4?
"AuthConfig.cs" configures security settings, including sites for OAuth login.
What is BundleConfig.cs in MVC 4?
"BundleConfig.cs" in MVC4 registers bundles used by the bundling and minification system. Several bundles are added by default, including jQuery, jQueryUI, jQuery validation, Modernizr, and default CSS references.
What is FilterConfig.cs in MVC 4?
This is used to register global MVC filters. The only filter registered by default is the HandleErrorAttribute, but this is a great place to put other filter registrations.
What is RouteConfig.cs in MVC 4?
"RouteConfig.cs" holds the granddaddy of the MVC config statements and Route configuration.
What is WebApiConfig.cs in MVC 4?
Used to register Web API routes, as well as set any additional Web API configuration settings.
What's new for adding a controller in a MVC 4 application?
Previously (in MVC 3 and MVC 2), the Visual Studio Add Controller menu item only displayed when we right-clicked on the Controllers folder. However, the use of the Controllers folder was purely for organization. (MVC will recognize any class that implements the IController interface as a Controller, regardless of its location in your application.) The MVC 4 Visual Studio tooling has been modified to display the Add Controller menu item for any folder in your MVC project. This allows us to organize controllers however you would like, perhaps separating them into logical groups or separating MVC and Web API controllers.
What are the software requirements of an ASP.NET MVC 4 application?
MVC 4 runs on the following Windows client operating systems:
- Windows XP
- Windows Vista
- Windows 7
- Windows 8
- Windows Server 2003
- Windows Server 2008
- Windows Server 2008 R2
What are the various types of Application Templates used to create an MVC application?
The various templates are as follows:
- The Internet Application template: This contains the beginnings of an MVC web application, enough that you can run the application immediately after creating it
and see a few pages. This template also includes some basic account management functions that run against the ASP.NET Membership.
- The Intranet Application template:
The Intranet Application template was added as part of the ASP.NET MVC 3
Tools Update. It is similar to the Internet Application template, but
the account management functions run against Windows accounts rather
than the ASP.NET Membership system.
- The Basic template:
This template is pretty minimal. It still has the basic folders, CSS,
and MVC application infrastructure in place, but no more. Running an
application created using the Empty template just gives you an error
message.
Why use Basic template? The Basic template is intended for experienced MVC developers who want to set up and configure things exactly how they want them.
- The Empty template:
The Basic Template was previously called the Empty Template, but
developers complained that it wasn't quite empty enough. With MVC 4, the
previous Empty
Template was renamed Basic, and the new Empty Template is about as empty as possible.
It has the assemblies and basic folder structure in place, but that's about it.
- The Mobile Application template: The
Mobile Application template is preconfigured with jQuery Mobile to
jump-start creating a mobile only website. It includes mobile visual
themes, a touch-optimized UI, and support for Ajax navigation.
- The Web API template: The ASP.NET Web API is a framework for creating HTTP services.
The Web API template is similar to the Internet Application template but is streamlined for Web API development. For instance, there is no user account management functionality, since Web API account management is often significantly different from standard MVC account management. Web API functionality is also available in the other MVC project templates, and even in non-MVC project types.
The default Top-level directories are:
- /Controllers: For Controller classes that handle URL requests
- /Models: For classes that represent and manipulate data and business objects
- /Views: For UI template files that are responsible for rendering output like HTML
- /Scripts: For JavaScript library files and scripts (.js)
- /Images: For images used in your site
- /Content: For CSS and other site content, other than scripts and images
- /Filters: For code filters
- /App_Data: To store data files you want to read/write
- /App_Start: For configuration code of features like Routing, Bundling, Web API.
ASP.NET MVC namespaces as well as classes are located in assembly System.Web.Mvc.
Note: Some of the content has been taken from various books/articles.
What is System.Web.Mvc namespace?
This namespace contains classes and interfaces that support the MVC pattern for ASP.NET Web applications. This namespace includes classes that represent controllers, controller
factories, action results, views, partial views, and model binders.
What is System.Web.Mvc.Ajax namespace?
The System.Web.Mvc.Ajax namespace contains classes that support Ajax scripting in an ASP.NET MVC application. The namespace includes support for Ajax scripts and Ajax option settings as well.
What is System.Web.Mvc.Async namespace?
The System.Web.Mvc.Async namespace contains classes and interfaces that support asynchronous actions in an ASP.NET MVC application.
What is System.Web.Mvc.Html namespace?
The System.Web.Mvc.Html namespace contains classes that help render HTML controls in an MVC application. This namespace includes classes that support forms, input controls, links, partial views, and validation.
What is ViewData, ViewBag and TempData?
MVC provides ViewData, ViewBag and TempData for passing data from the controller, view and in subsequent requests as well. ViewData and ViewBag are similar to some extent but TempData performs additional roles.
What are the roles and similarities between ViewData and ViewBag?
- Maintains data when moving from controller to view.
- Passes data from the controller to the respective view.
- Their value becomes null when any redirection occurs, because their role is to provide a way to communicate between controllers and views. It's a communication mechanism within the server call.
- ViewData is a dictionary of objects derived from the ViewDataDictionary class and accessible using strings as keys.
- ViewBag is a dynamic property that takes advantage of the new dynamic features in C# 4.0.
- ViewData requires typecasting for complex data types and checks for null values to avoid error.
- ViewBag doesn't require typecasting for complex data types.
One obvious difference is that ViewBag works only when the key being accessed is a valid C# identifier. For example, if you place a value in ViewData["KeyWith Spaces"] then you can't access that value using ViewBag because the code won't compile.
Another key issue to be aware of is that dynamic values cannot be passed in as parameters to extension methods. The C# compiler must know the real type of every parameter at compile time in order for it to choose the correct extension method.
If any parameter is dynamic then compilation will fail. For example, this code will always fail: @Html.TextBox("name", ViewBag.Name). To work around this, either use ViewData["Name"] or cast the value to a specifi c type: (string) ViewBag.Name.
What is TempData?
TempData is a dictionary derived from the TempDataDictionary class and stored in a short-lived session. It is a string key and object value.
It maintains the information for the duration of an HTTP Request. This means only from one page to another. It helps to maintain data when we move from one controller to another controller or from one action to another action. In other words, when we redirect Tempdata, it helps to maintain the data between those redirects. It internally uses session variables. Temp data used during the current and subsequent request only means it is used when we are sure that the next request will be redirecting to the next view. It requires typecasting for complex data types and checks for null values to avoid errors. Generally it is used to store only one-time messages, like error messages and validation messages.
How can you define a dynamic property using viewbag in ASP.NET MVC?
Assign a key name with the syntax "ViewBag.[Key]=[ Value]" and value using the equal to operator.
For example, you need to assign a list of students to the dynamic Students property of ViewBag as in the following:List<string> students = new List<string>();countries.Add("Akhil");countries.Add("Ekta");
ViewBag.Students = students;//Students is a dynamic property associated with ViewBag.
Note: Some of the content has been taken from various books/articles.
What is ViewModel (taken from stackoverflow)?
Accepted A view model represents data that you want to have displayed on your view/page.
Let's say that you have an Employee class that represents your employee domain model and it contains the following 4 properties:public class Employee : IEntity
{
public int Id { get; set; } // Employee's unique identifier public string FirstName { get; set; } // Employee's first name public string LastName { get; set; } // Employee's last name public DateTime DateCreated { get; set; } // Date when employee was created}
View models differ from domain models in that view models only contain the data (represented by properties) that you want to use on your view. For example, let's say that you want to add a new employee record, your view model might look like this:public class CreateEmployeeViewModel{
public string FirstName { get; set; }
public string LastName { get; set; }}
As you can see it only contains 2 of the properties of the employee domain model. Why is this you may ask? Id might not be set from the view and it might be auto-generated by the Employee table. And DateCreated might also be set in the Stored Procedure or in the service layer of your application. So Id and DateCreated is not needed in the view model.
When loading the view/page, the create action method in your employee controller will create an instance of this view model, populate any fields if required, and then pass this view model to the view:public class EmployeeController : Controller
{
private readonly IEmployeeService employeeService;
public EmployeeController(IEmployeeService employeeService)
{
this.employeeService = employeeService;
}
public ActionResult Create()
{
CreateEmployeeViewModel viewModel = new CreateEmployeeViewModel();
return View(viewModel);
}
public ActionResult Create(CreateEmployeeViewModel viewModel)
{
// Do what ever needs to be done before adding the employee to the database }}
Your view might look like this (assuming you are using ASP.NET MVC3 and razor):@model MyProject.Web.ViewModels.ProductCreateViewModel <table>
<tr>
<td><b>First Name:</b></td>
<td>@Html.TextBoxFor(x => x.FirstName, new { maxlength = "50", size = "50" })@Html.ValidationMessageFor(x => x.FirstName)</td>
</tr>
<tr>
<td><b>Last Name:</b></td>
<td>@Html.TextBoxFor(x => x.LastName, new { maxlength = "50", size = "50" })@Html.ValidationMessageFor(x => x.LastName)</td>
</tr></table>
Validation would thus be done only on FirstName and LastName. Using Fluent Validation you might have validation like this:
public class CreateEmployeeViewModelValidator : AbstractValidator<CreateEmployeeViewModel>
{
public CreateEmployeeViewModelValidator()
{
RuleFor(x => x.FirstName)
.NotEmpty()
.WithMessage("First name required")
.Length(1, 50)
.WithMessage("First name must not be greater than 50 characters");
RuleFor(x => x.LastName)
.NotEmpty()
.WithMessage("Last name required")
.Length(1, 50)
.WithMessage("Last name must not be greater than 50 characters");
}}
The key thing to remember is that the view model only represents the data that you want to use. You can imagine all the unnecessary code and validation if you have a domain model with 30 properties and you only want to update a single value. Given this scenario you would only have this one value/property in the view model and not the entire domain object.
How do you check for AJAX requests with C# in MVC.NET?
The solution is independed of the MVC.NET framework and is global across server side technologies. Most modern AJAX applications utilize XmlHTTPRequest to send async requests to the server. Such requests will have a distinct request header:
X-Requested-With = XMLHTTPREQUEST
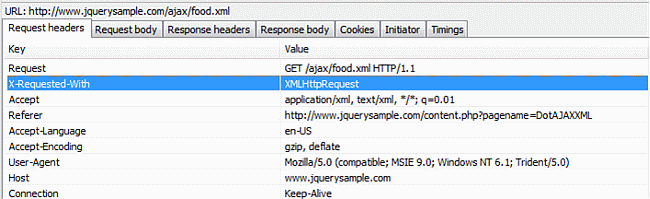
MVC.NET provides helper functions to check for ajax requests that internally inspects the "X-Requested-With" request header to set the "IsAjax" flag.
What are Scaffold templates?
These templates use the Visual Studio T4 templating system to generate a view based on the model type selected. Scaffolding in ASP.NET MVC can generate the boilerplate code we need to create, read, update, and delete (CRUD) functionality in an application. The scaffolding templates can examine the type definition for it then generate a controller and the controller's associated views. The scaffolding knows how to name controllers, how to name views, what code needs to go to each component, and where to place all these pieces in the project for the application to work.
What are the types of Scaffolding Templates?
- Empty: Creates an empty view. Only the model type is specified using the model syntax.
- Create: Creates a view with a form for creating new instances of the model.
Generates a label and input field for each property of the model type. - Delete: Creates a view with a form for deleting existing instances of the model.
Displays a label and the current value for each property of the model. - Details: Creates a view that displays a label and the value for each property of the model type.
- Edit: Creates a view with a form for editing existing instances of the model.
Generates a label and input field for each property of the model type. - List: Creates a view with a table of model instances. Generates a column for each property of the model type. Make sure to pass an IEnumerable<YourModelType> to this view from your action method.
Show an example of the difference in syntax in Razor and WebForm Views
Razor <span>@model.Message</span>Web Forms <span><%: model.Message %></span>
Code expressions in Razor are always HTML encoded. This Web Forms syntax also automatically HTML encodes the value.
What are Code Blocks in Views?
Unlike code expressions that are evaluated and sent to the response, blocks of code are simply sections of code that are executed. They are useful for declaring variables that we may need to use later.
Razor @{int x = 123;string y = Ëbecause.Ë;}
Web Forms
<%
int x = 123;
string y = "because.";
%>
What is the "HelperPage.IsAjax" Property?
The HelperPage.IsAjax property gets a value that indicates whether Ajax is being used during the request of the Web page.
Namespace: System.Web.WebPages
Assembly: System.Web.WebPages.dll
However, the same can be done by checking request headers directly:Request["X-Requested-With"] == "XmlHttpRequest".
Explain combining text and markup in Views using an example
This example shows what intermixing text and markup looks like using Razor as compared to Web Forms:
Razor @foreach (var item in items) {<span>Item @item.Name.</span>}
Web Forms
<% foreach (var item in items) { %>
<span>Item <%: item.Name %>.</span>
<% } %>
Explain Repository Pattern in ASP.NET MVC
In simple terms, a repository basically works as a mediator between our business logic layer and our data access layer of the application. Sometimes it would be troublesome to expose the data access mechanism directly to the business logic layer, it may result in redundant code for accessing data for similar entities or it may result in code that is hard to test or understand. To overcome these kinds of issues, and to write interface driven and test driven code to access data, we use the Repository Pattern. The repository makes queries to the data source for the data then maps the data from the data source to a business entity/domain object and finally persists the changes in the business entity to the data source. According to MSDN, a repository separates the business logic from the interactions with the underlying data source or Web Service. The separation between the data and business tiers has the following three benefits:
Code expressions in Razor are always HTML encoded. This Web Forms syntax also automatically HTML encodes the value.
What are Code Blocks in Views?
Unlike code expressions that are evaluated and sent to the response, blocks of code are simply sections of code that are executed. They are useful for declaring variables that we may need to use later.
Razor @{int x = 123;string y = Ëbecause.Ë;}
Web Forms
<%
int x = 123;
string y = "because.";
%>
What is the "HelperPage.IsAjax" Property?
The HelperPage.IsAjax property gets a value that indicates whether Ajax is being used during the request of the Web page.
Namespace: System.Web.WebPages
Assembly: System.Web.WebPages.dll
However, the same can be done by checking request headers directly:Request["X-Requested-With"] == "XmlHttpRequest".
Explain combining text and markup in Views using an example
This example shows what intermixing text and markup looks like using Razor as compared to Web Forms:
Razor @foreach (var item in items) {<span>Item @item.Name.</span>}
Web Forms
<% foreach (var item in items) { %>
<span>Item <%: item.Name %>.</span>
<% } %>
Explain Repository Pattern in ASP.NET MVC
In simple terms, a repository basically works as a mediator between our business logic layer and our data access layer of the application. Sometimes it would be troublesome to expose the data access mechanism directly to the business logic layer, it may result in redundant code for accessing data for similar entities or it may result in code that is hard to test or understand. To overcome these kinds of issues, and to write interface driven and test driven code to access data, we use the Repository Pattern. The repository makes queries to the data source for the data then maps the data from the data source to a business entity/domain object and finally persists the changes in the business entity to the data source. According to MSDN, a repository separates the business logic from the interactions with the underlying data source or Web Service. The separation between the data and business tiers has the following three benefits:
- It centralizes the data logic or Web service access logic.
- It provides a substitution point for the unit tests.
- It provides a flexible architecture that can be adapted as the overall design of the application evolves.
In
a Repository we write our entire business logic of CRUD operations
using Entity Framework classes that will not only result in meaningful
test driven code but will also reduce our controller code of accessing
data.
How can you call a JavaScript function/method on the change of a Dropdown List in MVC?
Create a JavaScript method:<script type="text/javascript"> function selectedIndexChanged() {
}</script>
Invoke the method:
<%:Html.DropDownListFor(x => x.SelectedProduct,
new SelectList(Model.Users, "Value", "Text"),
"Please Select a User", new { id = "ddlUsers",
onchange="selectedIndexChanged()" })%>
Explain Routing in MVC
A route is a URL pattern that is mapped to a handler. The handler can be a physical file, such as an .aspx file in a Web Forms application. A Routing module is responsible for mapping incoming browser requests to specific MVC controller actions.
Routing within the ASP.NET MVC framework serves the following two main purposes:
How can you call a JavaScript function/method on the change of a Dropdown List in MVC?
Create a JavaScript method:<script type="text/javascript"> function selectedIndexChanged() {
}</script>
Invoke the method:
<%:Html.DropDownListFor(x => x.SelectedProduct,
new SelectList(Model.Users, "Value", "Text"),
"Please Select a User", new { id = "ddlUsers",
onchange="selectedIndexChanged()" })%>
Explain Routing in MVC
A route is a URL pattern that is mapped to a handler. The handler can be a physical file, such as an .aspx file in a Web Forms application. A Routing module is responsible for mapping incoming browser requests to specific MVC controller actions.
Routing within the ASP.NET MVC framework serves the following two main purposes:
- It matches incoming requests that would not otherwise match a file on the file system and maps the requests to a controller action.
- It constructs outgoing URLs that correspond to controller actions.
How is a route table created in ASP.NET MVC?
When an MVC application first starts, the Application_Start() method in global.asax is called. This method calls the RegisterRoutes() method. The RegisterRoutes() method creates the route table for the MVC application.
What are Layouts in ASP.NET MVC Razor?
Layouts in Razor help maintain a consistent look and feel across multiple views within our application. Compared to Web Forms Web Forms, layouts serve the same purpose as master pages, but offer both a simpler syntax and greater flexibility.
We can use a layout to define a common template for your site (or just part of it). This template contains one or more placeholders that the other views in your application provide content for. In some ways, it's like an abstract base class for your views. For example declared at the top of view as in the following:@{Layout = "~/Views/Shared/SiteLayout.cshtml";}
What is ViewStart?
For group of views that all use the same layout, this can get a bit redundant and harder to maintain.
The "_ViewStart.cshtml" page can be used to remove this redundancy. The code within this file is executed before the code in any view placed in the same directory. This file is also recursively applied to any view within a subdirectory.
When we create a default ASP.NET MVC project, we find there is already a "_ViewStart .cshtml" file in the Views directory. It specifies a default layout as in the following:@{Layout = "~/Views/Shared/_Layout.cshtml";}
Because this code runs before any view, a view can override the Layout property and choose a different one. If a set of views shares common settings then the "_ViewStart.cshtml" file is a useful place to consolidate these common view settings. If any view needs to override any of the common settings then the view can set those values to another value.
Note: Some of the content has been taken from various books/articles.
What are HTML Helpers?
HTML helpers are methods we can invoke on the Html property of a view. We also have access to URL helpers (via the URL property) and AJAX helpers (via the Ajax property). All
these helpers have the same goal, to make views easy to author. The URL helper is also available from within the controller.
Most of the helpers, particularly the HTML helpers, output HTML markup. For example, the BeginForm helper is a helper we can use to build a robust form tag for our search
form, but without using lines and lines of code:@using (Html.BeginForm("Search", "Home", FormMethod.Get)) {<input type="text" name="q" />
<input type="submit" value="Search" />}
What is Html.ValidationSummary?
The ValidationSummary helper displays an unordered list of all validation errors in the ModelState dictionary. The Boolean parameter you are using (with a value of true) is telling the helper to exclude property-level errors. In other words, you are telling the summary to display only the errors in ModelState associated with the model itself, and exclude any errors associated with a specific model property. We will be displaying property-level errors separately. Assume you have the following code somewhere in the controller action rendering the edit view:
ModelState.AddModelError("", "This is all wrong!");
ModelState.AddModelError("Title", "What a terrible name!");
The first error is a model-level error, because you didn't provide a key (or provided an empty key) to associate the error with a specific property. The second error you associated with the Title property, so in your view it will not display in the validation summary area (unless you remove the parameter to the helper method, or change the value to false). In this scenario, the helper renders the following HTML:<div class="validation-summary-errors">
<ul>
<li>This is all wrong!</li>
</ul>
</div>
Other overloads of the ValidationSummary helper enable you to provide header text and set specific HTML attributes.
NOTE: By convention, the ValidationSummary helper renders the CSS class validation-summary-errors along with any specific CSS classes you provide. The default MVC project template includes some styling to display these items in red, that you can change in "styles.css".
What are Validation Annotations?
Data annotations are attributes you can find in the "System.ComponentModel.DataAnnotations" namespace. These attributes provide server-side validation, and the framework also supports client-side validation when you use one of the attributes on a model property. You can use four attributes in the DataAnnotations namespace to cover the common validation scenarios, Required, String Length, Regular Expression and Range.
What is Html.Partial?
The Partial helper renders a partial view into a string. Typically, a partial view contains reusable markup you want to render from inside multiple different views. Partial has four overloads:public void Partial(string partialViewName);public void Partial(string partialViewName, object model);public void Partial(string partialViewName, ViewDataDictionary viewData);public void Partial(string partialViewName, object model,
ViewDataDictionary viewData);
What is Html.RenderPartial?
The RenderPartial helper is similar to Partial, but RenderPartial writes directly to the response output stream instead of returning a string. For this reason, you must place RenderPartial inside a code block instead of a code expression. To illustrate, the following two lines of code render the same output to the output stream:@{Html.RenderPartial("AlbumDisplay "); }
@Html.Partial("AlbumDisplay ")
If they are same then which one to use?
In general, you should prefer Partial to RenderPartial because Partial is more convenient (you don't need to wrap the call in a code block with curly braces). However, RenderPartial may result in better performance because it writes directly to the response stream, although it would require a lot of use (either high site traffic or repeated calls in a loop) before the difference would be noticeable.
How do you return a partial view from controller?
When an MVC application first starts, the Application_Start() method in global.asax is called. This method calls the RegisterRoutes() method. The RegisterRoutes() method creates the route table for the MVC application.
What are Layouts in ASP.NET MVC Razor?
Layouts in Razor help maintain a consistent look and feel across multiple views within our application. Compared to Web Forms Web Forms, layouts serve the same purpose as master pages, but offer both a simpler syntax and greater flexibility.
We can use a layout to define a common template for your site (or just part of it). This template contains one or more placeholders that the other views in your application provide content for. In some ways, it's like an abstract base class for your views. For example declared at the top of view as in the following:@{Layout = "~/Views/Shared/SiteLayout.cshtml";}
What is ViewStart?
For group of views that all use the same layout, this can get a bit redundant and harder to maintain.
The "_ViewStart.cshtml" page can be used to remove this redundancy. The code within this file is executed before the code in any view placed in the same directory. This file is also recursively applied to any view within a subdirectory.
When we create a default ASP.NET MVC project, we find there is already a "_ViewStart .cshtml" file in the Views directory. It specifies a default layout as in the following:@{Layout = "~/Views/Shared/_Layout.cshtml";}
Because this code runs before any view, a view can override the Layout property and choose a different one. If a set of views shares common settings then the "_ViewStart.cshtml" file is a useful place to consolidate these common view settings. If any view needs to override any of the common settings then the view can set those values to another value.
Note: Some of the content has been taken from various books/articles.
What are HTML Helpers?
HTML helpers are methods we can invoke on the Html property of a view. We also have access to URL helpers (via the URL property) and AJAX helpers (via the Ajax property). All
these helpers have the same goal, to make views easy to author. The URL helper is also available from within the controller.
Most of the helpers, particularly the HTML helpers, output HTML markup. For example, the BeginForm helper is a helper we can use to build a robust form tag for our search
form, but without using lines and lines of code:@using (Html.BeginForm("Search", "Home", FormMethod.Get)) {<input type="text" name="q" />
<input type="submit" value="Search" />}
What is Html.ValidationSummary?
The ValidationSummary helper displays an unordered list of all validation errors in the ModelState dictionary. The Boolean parameter you are using (with a value of true) is telling the helper to exclude property-level errors. In other words, you are telling the summary to display only the errors in ModelState associated with the model itself, and exclude any errors associated with a specific model property. We will be displaying property-level errors separately. Assume you have the following code somewhere in the controller action rendering the edit view:
ModelState.AddModelError("", "This is all wrong!");
ModelState.AddModelError("Title", "What a terrible name!");
The first error is a model-level error, because you didn't provide a key (or provided an empty key) to associate the error with a specific property. The second error you associated with the Title property, so in your view it will not display in the validation summary area (unless you remove the parameter to the helper method, or change the value to false). In this scenario, the helper renders the following HTML:<div class="validation-summary-errors">
<ul>
<li>This is all wrong!</li>
</ul>
</div>
Other overloads of the ValidationSummary helper enable you to provide header text and set specific HTML attributes.
NOTE: By convention, the ValidationSummary helper renders the CSS class validation-summary-errors along with any specific CSS classes you provide. The default MVC project template includes some styling to display these items in red, that you can change in "styles.css".
What are Validation Annotations?
Data annotations are attributes you can find in the "System.ComponentModel.DataAnnotations" namespace. These attributes provide server-side validation, and the framework also supports client-side validation when you use one of the attributes on a model property. You can use four attributes in the DataAnnotations namespace to cover the common validation scenarios, Required, String Length, Regular Expression and Range.
What is Html.Partial?
The Partial helper renders a partial view into a string. Typically, a partial view contains reusable markup you want to render from inside multiple different views. Partial has four overloads:public void Partial(string partialViewName);public void Partial(string partialViewName, object model);public void Partial(string partialViewName, ViewDataDictionary viewData);public void Partial(string partialViewName, object model,
ViewDataDictionary viewData);
What is Html.RenderPartial?
The RenderPartial helper is similar to Partial, but RenderPartial writes directly to the response output stream instead of returning a string. For this reason, you must place RenderPartial inside a code block instead of a code expression. To illustrate, the following two lines of code render the same output to the output stream:@{Html.RenderPartial("AlbumDisplay "); }
@Html.Partial("AlbumDisplay ")
If they are same then which one to use?
In general, you should prefer Partial to RenderPartial because Partial is more convenient (you don't need to wrap the call in a code block with curly braces). However, RenderPartial may result in better performance because it writes directly to the response stream, although it would require a lot of use (either high site traffic or repeated calls in a loop) before the difference would be noticeable.
How do you return a partial view from controller?
return PartialView(options); //options could be Model or View name
What are various ways of returning a View?
There are various ways for returning/rendering a view in MVC Razor. For example "return View()", "return RedirectToAction()", "return Redirect()" and "return RedirectToRoute()".
What are various ways of returning a View?
There are various ways for returning/rendering a view in MVC Razor. For example "return View()", "return RedirectToAction()", "return Redirect()" and "return RedirectToRoute()".
What is a View Engine?
Ans:- View Engines are responsible for rendering the HTML from your views to the browser. The view engine template will have different syntax for implementation.
What is Razor view engine?
Ans:- The Razor view engine is an advanced view engine from Microsoft, It is launched with MVC 3 (in VS 4.0). Razor using an @ character instead classic ASP.NET(.aspx) <% %>
and Razor does not require you to explicitly close the code-block, this view engine is parsed intelligently by the run-time to determine what is a presentation element and what is a code element.
What are the two popular asp.net mvc view engines?
Ans:- 1. Razor
2. .aspx
What are the file extension for Razore view engine files?
Ans:- Razor syntax have the special file extension cshtml (Razor with C#) and vbhtml (Razor with VB).
Can you give a simple example of textbox?
Ans:- @Html.TextBox("Name")
What HTML code will be produce by "@Html.TextBox("Name")"?
Ans:- It will produce:-
<input id="Name" name="Name" type="textbox" />
What is the difference between @Html.TextBox and @Html.TextBoxFor
Ans:- Finaly both produce the same HTML but Html.TextBoxFor() is strongly typed with any model, where as Html.TextBox isn't.
TextBoxFor input extension is first time introduce in which MVC version?
Ans:- In MVC2
How to add Namespaces in Razor view engine?
Ans:- @using YourCustomNamespace
Linq stands for Language Integrated Query
A query is an expression that retrieves data from a data source. Queries are usually expressed in a specialized query language.
Microsoft language developers provided a way to express queries directly in their languages (such as c# and vb).
A query is an expression that retrieves data from a data source. Queries are usually expressed in a specialized query language.
Three Parts of a Query Operation
All LINQ query operations consist of three distinct actions:
-
Obtain the data source.
-
Create the query.
-
Execute the query.
- Linq To Objects - examine System.Linq.Enumerable for query methods. These target
IEnumerable<T>
, allowing any typed loopable collection to be queried in a type-safe manner. These queries rely on compiled .Net methods, not Expressions. - Linq To Anything - examine System.Linq.Queryable for some query methods. These target
IQueryable<T>
, allowing the construction of Expression Trees that can be translated by the underlying implementation. - Expression Trees - examine System.Linq.Expressions namespace. This is code as data. In practice, you should be aware of this stuff, but don't really need to write code against these types. Language features (such as lambda expressions) can allow you to use various short-hands to avoid dealing with these types directly.
- Linq To Sql - examine the System.Data.Linq namespace. Especially note the
DataContext
. This is a DataAccess technology built by the C# team. It just works. - Linq To Entities - examine the System.Data.Objects namespace. Especially note the
ObjectContext
. This is a DataAccess technology built by the ADO.Net team. It is complex, powerful, and harder to use than Linq To Sql. - Linq To Xml - examine the System.Xml.Linq namespace. Essentially, people weren't satisfied with the stuff in
System.Xml
. So MS re-wrote it and took advantage of the re-write to introduce some methods that make it easier to use LinqToObjects against Xml.
LINQ to SQL is one of the most widely used implementation of LINQ. It is so common that many developers completely overlooked other implementations of LINQ such as LINQ to DataSet or LINQ to Objects. Although LINQ to SQL is only available for SQL Server databases but it is still using all the features available in the other LINQ implementations. In this tutorial, I will give you brief introduction of LINQ to SQL, DataContext object, Entity classes and Object Relational Designer along with some simple LINQ to SQL queries.In the current world of object-oriented programming languages such as C# or VB.NET, developers model their classes to represent real world object such as employees, customers, orders etc. These objects need to be persistent in such a way that their data should not be lost when the application close or restart. However, most of the databases used these days are relational and they store data as records in tables, not as objects. This caused a mismatch between the object-oriented world and the relational database world. For example, an employee class that contains multiple addresses or contact numbers stored in collections will most likely be stored in multiple database tables such as employee table, an address and contacts table.
Another problem is that the data types in relational database are normally different than the data types available in object-oriented programming languages. To read data from databases into programs, developers need to do lot of data types conversions which not only required extra work but also error prone.
LINQ to SQL is an API for working with SQL Server databases. It translates LINQ expressions to equivalent SQL or T-SQL queries and passes them on to the SQL Server database for execution and then returns the results back to the calling application. To solve the problem of mismatching between database tables and objects, LINQ to SQL generates object-relational mapping (ORM) implementation to seamlessly maps tables and columns to classes and properties with the help of mapping attributes. One can argue that there are already hundreds of ORM tools in the market that do exactly what LINQ does, mean they can also generate the abstract layer of business objects mapped with database tables. But you have to keep in mind that those tools does not provide you the full blown query language that is similar to SQL and also integrated directly into the programming language as LINQ does.
LINQ to SQL Data Modeling
To use LINQ to SQL in programs developers need to create a thin abstraction layer over the relational database model. This layer contains set of entity classes which are mapped to tables in databases. By using these entity classes the data in tables can not only be queried but can also be modified. There are two ways you can generate these entity classes in your project.
Object Relational Designer
Object Relational Designed also called O/R Designer provides an easy to use graphical interface for creating entity classes one at a time. You can add or remove database tables directly to the designer and the corresponding entity classes will be generated automatically for you.
SQLMetal
SQLMetal is a command line tool to generate entity classes for all the tables, views, stored procedures at once. This tool is available at Visual Studio command prompt and if you will just type sqlmetal in command window you will see all the available options for this tool.
The DataContext
The DataContext class handles the communication between LINQ and external relational database. Each instance of this class establishes a connection to a database and provides several services such as identity tracking, change tracking etc. In LINQ to SQL context, the DataContext class connects us to the database, monitor what we have changed and update the database when needed by the program. To use this class in your application, you typically need to create a class derived from the DataContext class and normally it has the same name as your database. If you are using SQLMetal tool described above, it will also generates the DataContext child class with the same name as your database.
The DataContext class uses metadata information to map the physical structure of relational data on which the entity classes and the code generation is based. Once you have the class derived from the DataContext class, it is easier to create entity classes as members of this class to represent the tables in underlying database.
To explain you how all these important concepts work together lets create a Visual Studio project and use Object Relational Designer to generate the DataContext and entity classes. For the purpose of this tutorial, I have created a sample database named SampleDB in SQL Server 2005 Express Edition with the two tables shown in the figure below. I have also added some records in the tables to perform LINQ to SQL queries later in this tutorial.
You will see the Object Relational Designer appears inside Visual Studio asking you to create data classes by dragging items from Server Explorer or Toolbox to the design surface. You need to add a database connection inside Server Explorer to drag database tables to the designer. Make sure your Server Explorer is visible and if your Server Explorer is not visible go to View menu and click Server Explorer. Inside Server Explorer right click on Data Connections and click Add Connection option. You will see Add Connection dialog box appearing on screen asking you information about your data source. For this tutorial I have given the information about my database as shown in the figure below. You can see the SampleDB is selected as a database name to generate the DataContext class. You can also click the button Test Connection to check whether the information you have provided is correct or not. Click the OK button once you have done with Add Connection dialog box.
Once the connection is added in Server Explorer, you need to expand the Tables collection in the database and need to drag the Categories and Products table to the designer surface as shown in the figure below:
Save and close the SampleDB.dbml file and locate the file named SampleDB.designer.cs in the Solution Explorer. I want you to check the DataContext derived class and entity classes generated for you automatically by the Object Relational Designer in this file. The first line of interest is the following class declaration in which you can see how the class named SampleDBDataContext is mapped to my SampleDB database with the help of DatabaseAttribute. You can also see the class is inheriting the System.Data.Linq.DataContext class.
[System.Data.Linq.Mapping.DatabaseAttribute(Name="SampleDB")]
public partial class SampleDBDataContext : System.Data.Linq.DataContext
{
}
The next important piece of code in the class is automatically generated nested class named Category. This is an entity class which is mapped to the Categories table in the database and you can guess it by looking at the Table attribute on top of the class. The similar code is also generated for the Products table with another entity class named Product.
[Table(Name="dbo.Categories")]
public partial class Category : INotifyPropertyChanging, INotifyPropertyChanged
{
}
Inside the generated entity classes you can also see how the columns in database tables are mapped to class properties. For example the
CategoryName column in database table is mapped with the help of Column attribute as shown in the code below:
[Column(Storage="_CategoryName", DbType="NVarChar(50) NOT NULL", CanBeNull=false)]
public string CategoryName
{
}
You can see how easy is to generate a complete ORM abstraction layer with the help of Object Relational Designer. Once the DataContext and entity classes are generated you are ready to run LINQ to SQL queries to your underlying database. The remaining of this tutorial will show you how to run some simple LINQ to SQL queries using the DataContext, entity classes and LINQ to SQL operators.
The first thing you need before running LINQ to SQL queries is the database connection string. You can store it your project configuration file but for this tutorial I am saving it directly in a local string variable. Next you need to create the SampleDBDataContext object and need to pass your connection string into its constructor as shown below:
string constr = @"Server=Waqas\SQLEXPRESS; Database=SampleDB; uid=sa; pwd=123;";
SampleDBDataContext db = new SampleDBDataContext(constr);
Now I am creating a query to display all the categories from the Categories table in my database. I am also doing sorting based on the CategoryID column with the help of OrderBy operator.
var query = from c in db.Categories
orderby c.CategoryID
select c;
foreach (var item in query)
{
Console.WriteLine(item.CategoryID + " : " + item.CategoryName);
} If you will run the above query you will see the results similar to the following output depending upon the data you have in your categories table in database.
where p.UnitPrice > 300
orderby p.UnitPrice
select p;
foreach (var item in query)
{
Console.WriteLine(item.UnitPrice + " : " + item.ProductName);
}
The above query will display the following results when executed.
You can perform all types of queries now on your database tables with the help of hundred of operators available in LINQ. You can perform complex multi table joins or can perform grouping on your tables. You can also insert, update or delete data in your tables with the help of LINQ to SQL queries as well as you can perform complex multi table updates with the help of transactions support available in LINQ. It is not possible for me to cover all these things in this tutorial but I will be writing more tutorials on these topics very soon and will published those tutorials for all of you on my website. I hope I have given you enough information about LINQ to SQL in this tutorial that you can dig deeper into the world of LINQ to SQL yourself and can play more tricks with LINQ in your applications.
What are the advantages of hosting WCF Services in IIS as
compared to self-hosting?
There are two main advantages of using IIS over self-hosting:-
Automatic activation
IIS provides automatic activation that means the service is not necessary to be running in
advance. When any message is received by the service it then launches and fulfills the request.
But in case of self hosting the service should always be running.
Process recycling
If IIS finds that a service is not healthy that means if it has memory leaks etc, IIS recycles the
process.
For every browser instance, a worker process is spawned and the request is serviced. When the browser disconnects the worker,
process stops and you loose all information. IIS also restarts the worker process. By default, the
worker process is recycled at around 120 minutes. So why does IIS recycle. By restarting the
worker process it ensures any bad code or memory leak do not cause issue to the whole system.
In case of self-hosting both the above features, you will need to code yourself. Lot of work
right!!.
what are the various ways of hosting a WCF service?
There are three major ways to host a WCF service:-
• Self-hosting the service in his own application domain. This we have already covered in
the first section. The service comes in to existence when you create the object of Service
Host class and the service closes when you call the Close of the Service Host class.
• Host in application domain or process provided by IIS Server.
• Host in Application domain and process provided by WAS (Windows Activation
Service) Server.
What is WCF?
WCF stands for Windows Communication Foundation. It is a Software development kit for developing services on Windows. WCF is introduced in .NET 3.0. in the System.ServiceModel namespace. WCF is based on basic concepts of Service oriented architecture (SOA)
It consists of three main points: Address,Binding and Contract.
Windows Communication Foundation (WCF) is an SDK for developing and deploying services on Windows. WCF provides a runtime environment for services, enabling you to expose CLR types as services, and to consume other services as CLR types.
WCF is part of .NET 3.0 and requires .NET 2.0, so it can only run on systems that support it
WCF(Indigo was the code name for WCF) is a unification of .Net framework communication technologies.
Endpoint
Every service must have Address that defines where the service resides, Contract that defines what the service does and a Binding that defines how to communicate with the service. In WCF the relationship between Address, Contract and Binding is called Endpoint.
The Endpoint is the fusion of Address, Contract and Binding.
Explain Address,Binding and contract for a WCF Service?
Address: Address defines where the service resides.
Binding: Binding defines how to communicate with the service.
Contract: Contract defines what is done by the service.
Address
Address is a way of letting client know that where a service is located. In WCF, every service is associated with a unique address. This contains the location of the service and transport schemas.
WCF supports following transport schemas
HTTP
TCP
Peer network
IPC (Inter-Process Communication over named pipes)
MSMQ
The sample address for above transport schema may look like
http://localhost:81
http://localhost:81/MyService
net.tcp://localhost:82/MyService
net.pipe://localhost/MyPipeService
net.msmq://localhost/private/MyMsMqService
net.msmq://localhost/MyMsMqService
Bindings
A binding defines how an endpoint communicates to the world. A binding defines the transport (such as HTTP or TCP) and the encoding being used (such as text or binary). A binding can contain binding elements that specify details like the security mechanisms used to secure messages, or the message pattern used by an endpoint.
A binding defines how an endpoint communicates to the world. A binding defines the transport (such as HTTP or TCP) and the encoding being used (such as text or binary). A binding can contain binding elements that specify details like the security mechanisms used to secure messages, or the message pattern used by an endpoint.
WCF supports nine types of bindings.
Basic binding
Offered by the BasicHttpBinding class, this is designed to expose a
WCF service as a legacy ASMX web service, so that old clients can work
with new services. When used by the client, this binding enables new
WCF clients to work with old ASMX services.
TCP binding
Offered by the NetTcpBinding class, this uses TCP for cross-machine
communication on the intranet. It supports a variety of features,
including reliability, transactions, and security, and is optimized for
WCF-to-WCF communication. As a result, it requires both the client and
the service to use WCF.
Peer network binding
Offered by the NetPeerTcpBinding class, this uses peer networking as a transport. The peer network-enabled client and services all subscribe to the same grid and broadcast messages to it.
IPC binding
Offered by the NetNamedPipeBinding class, this uses named pipes as
a transport for same-machine communication. It is the most secure
binding since it cannot accept calls from outside the machine and it
supports a variety of features similar to the TCP binding.
Web Service (WS) binding
Offered by the WSHttpBinding class, this uses HTTP or HTTPS for
transport, and is designed to offer a variety of features such as
reliability, transactions, and security over the Internet.
Federated WS binding
Offered by the WSFederationHttpBinding class, this is a
specialization of the WS binding, offering support for federated
security.
Duplex WS binding
Offered by the WSDualHttpBinding class, this is similar to the WS
binding except it also supports bidirectional communication from the
service to the client.
MSMQ binding
Offered by the NetMsmqBinding class, this uses MSMQ for transport
and is designed to offer support for disconnected queued calls.
MSMQ integration binding
Offered by the MsmqIntegrationBinding class, this converts WCF
messages to and from MSMQ messages, and is designed to interoperate
with legacy MSMQ clients.Contracts
In WCF, all services expose contracts. The contract is a platform-neutral and standard way of describing what the service does.
WCF defines four types of contracts.
Service contracts
Describe which operations the client can perform on the service.
There are two types of Service Contracts.
ServiceContract - This attribute is used to define the Interface.
OperationContract
This attribute is used to define the method inside Interface.
[ServiceContract] interface IMyContract { [OperationContract] string MyMethod( ); } class MyService : IMyContract { public string MyMethod( ) { return "Hello World"; } }
Data contracts
Define which data types are passed to and from the service. WCF defines implicit contracts for built-in types such as int and string, but we can easily define explicit opt-in data contracts for custom types.
There are two types of Data Contracts.
DataContract - attribute used to define the class
DataMember - attribute used to define the properties.
[DataContract] class Contact { [DataMember] public string FirstName; [DataMember] public string LastName; }
If DataMember attributes are not specified for a properties in the class, that property can't be passed to-from web service.
Fault contracts
Define which errors are raised by the service, and how the service handles and propagates errors to its clients.
Message contracts
Allow the service to interact directly with messages. Message contracts can be typed or untyped, and are useful in interoperability cases and when there is an existing message format we have to comply with.
Where we Can host WCS Services
Every WCF services must be hosted somewhere. There are three ways of hosting WCF services.
1. IIS
2. Self Hosting
3. WAS (Windows Activation Service)
How to define a service as REST based service in WCF?
WCF 3.5 provides explicit support for RESTful communication using a new binding named WebHttpBinding.
The below code shows how to expose a RESTful service
[ServiceContract] interface IStock { [OperationContract] [WebGet] int GetStock(string StockId); }By adding the WebGetAttribute, we can define a service as REST based service that can be accessible using HTTP GET operation.
What are the main components of WCF? | |
---|---|
|
What are the advantages of hosting WCF Services in IIS as compared to self hosting?
- There are two main advantages of using IIS over self hosting.
- Automatic activation
- Process recycling
Web services can only be invoked by HTTP. While Services or a WCF component can be invoked by any protocol and any transport type. Second web services are not flexible. However Services are flexible. If you make a new version of the service then you need to just expose a new end. Therefore services are agile and which is a very practical approach looking at the current business trends.
Can we have two way communications in MSMQ?
Yes
What are dead letter queues?
The main use of queue is that you do not need the client and the server
running at one time. Therefore it is possible that a message will lie
in queue for long time until the server or client picks it up. But
there are scenarios where a message is no use after a certain time.
what are the various ways of hosting a WCF service?
- There are three major ways to host a WCF service :
- Self-hosting the service in his own application domain. This we have already covered in the first section. The service comes in to existence when you create the object of Service Host class and the service closes when you call the Close of the Service Host class.
- Host in application domain or process provided by IIS Server.
- Host in Application domain and process provided by WAS (Windows Activation Service) Server
SOA is a collection of well defined services, where each individual service can be modified independently of other services to help respond to the ever evolving market conditions of a business.
What is the difference between transport level and message level security?
- Transport level security happens at the channel level. Transport level security is the easiest to implement as it happens at the communication level. WCF uses transport protocols like TCP, HTTP, MSMQ etc and every of these protocols have their own security mechanisms.
- Message level security is implemented with message data itself.
What is service and client in perspective of data communication?
|
- WCF is interoperable with other services when compared to .Net Remoting,where the client and service have to be .Net.
- WCF services provide better reliability and security in compared to ASMX web services.
- In WCF, there is no need to make much change in code for implementing the security model and changing the binding.
- WCF has integrated logging mechanism, changing the configuration file settings will provide this functionality.
What are the types of bindings in WCF?
- Basic binding
- TCP binding
- Peer network binding
- IPC binding
- Web Service (WS) binding
- Federated WS binding
- Duplex WS binding
- MSMQ binding
- MSMQ integration binding
WCF Services client configuration file contains endpoint, address, binding and contract.
What are the features of WCF?
- Transactions : A transaction is a unit of work. A transaction ensures that everything within that transaction either succeeds as a whole or fails as whole.
- Hosting : WCF hosting allows services to be hosted in a handful of different environments, such as Windows NT Services, Windows Forms, and console applications, and well as IIS (Internet Information Services) and Windows Activation Services (WAS).
- Security : WCF also enables you to integrate your application into an existing security infrastructure, including those that extend beyond the standard Windows-only environments by using secure SOAP messages.
- Queuing : WCF enables queuing by providing support for the MSMQ transport.
What are the main components of WCF?
- The main components of WCF are :
- Service class
- Hosting environment
- End point
What are the types of messaging patterns?
There are three basic messaging patterns that programs can use to exchange messages. - Simplex
- Duplex
- Request Reply
WCF EXAMPLE-1
WCF EXAMPLE-2
WCF EXAMPLE-3
MVC
The business layer (Model logic)
The display layer (View logic)
The input control (Controller logic)
MVC is one of three ASP.NET programming models.
MVC is a framework for building web applications using a MVC (Model View Controller) design:
- The Model represents the application core (for instance a list of database records).
- The View displays the data (the database records).
- The Controller handles the input (to the database records).
Web Forms vs MVC
The MVC programming model is a lighter alternative to traditional ASP.NET (Web Forms).
It is a lightweight, highly testable framework, integrated
with all existing ASP.NET features, such as Master Pages, Security, and
Authentication.The Model-View-Controller (MVC) pattern separates the modeling of the domain, the presentation, and the actions based on user input into three separate classes [Burbeck92]:
Model–view–controller (MVC) is a software architecture pattern which separates the representation of information from the user's interaction with it.The model consists of application data, business rules, logic, and functions. A view can be any output representation of data, such as a chart or a diagram. Multiple views of the same data are possible, such as a bar chart for management and a tabular view for accountants. The controller mediates input, converting it to commands for the model or view. The central ideas behind MVC are code reusability and separation of concerns
- A controller can send commands to its associated view to change the view's presentation of the model (e.g., by scrolling through a document). It can also send commands to the model to update the model's state (e.g., editing a document).
- A model notifies its associated views and controllers when there has been a change in its state. This notification allows the views to produce updated output, and the controllers to change the available set of commands. A passive implementation of MVC omits these notifications, because the application does not require them or the software platform does not support them.[6]
- A view requests from the model the information that it needs to generate an output representation to the user.gfhgfhfghgf
Client-Side
Validation Templated Helpers Areas Asynchronous Controllers
Html.ValidationSummary Helper Method DefaultValueAttribute in Action-Method
Parameters Binding Binary Data with Model Binders DataAnnotations Attributes
Model-Validator Providers New RequireHttpsAttribute Action Filter Templated
Helpers Display Model-Level Errors
|
Razor
Readymade project templates
HTML 5 enabled templatesSupport for
Multiple View EnginesJavaScript and Ajax
Model Validation Improvements
|
ASP.NET
Web API
Refreshed and modernized default
project
Templates New mobile project
template
Many new features to support
mobile apps
Enhanced support for asynchronous
methods
|
Razor view
Razor is not a new programming language itself, but uses C# or
Visual Basic syntax for having code inside a page without ASP.NET
delimiter: <%= %>. Razor file extension is ‘cshtml’ for C# language, and ‘vbhtml’ for Visual Basic.
Syntax
Before
getting introduced to Razor you should first know some simple rules
that will help you understand how to write HTML with C# or Visual Basic
in the same page:- ‘@’ is the magic character that precedes code instructions in the following contexts:
- ‘@’ For a single code line/values:A single code line inside the markup:
cshtml<p> Current time is: @DateTime.Now </p>
vbhtml<p> Current time is: @DateTime.Now </p>
- ‘@{ … }’ For code blocks with multiple lines:cshtml
@{ var name = “John”; var nameMessage = "Hello, my name is " + name + " Smith"; }
vbhtml@Code Dim name = “John” Dim nameMessage = "Hello, my name is " + name + " Smith" End Code
- ‘@:’ For single plain text to be rendered in the page.cshtml
@{ @:The day is: @DateTime.Now.DayOfWeek. It is a <b>great</b> day! }
vbhtml@Code @:The day is: @DateTime.Now.DayOfWeek. It is a <b>great</b> day! End Code
- ‘@’ For a single code line/values:A single code line inside the markup:
- HTML markup lines can be included at any part of the code:It
is no need to open or close code blocks to write HTML inside a page. If
you want to add a code instruction inside HTML, you will need to use
‘@’ before the code:
cshtml@if(IsPost) { <p>Hello, the time is @DateTime.Now and this page is a postback!</p> } else { <p>Hello, today is: </p> @DateTime.Now }
vbhtml@If IsPost Then @<p>Hello, the time is @DateTime.Now and this page is a postback!</p> Else @<p>Hello, today is: </p> @DateTime.Now End If
- Razor uses code syntax to infer indent:Razor
Parser infers code ending by reading the opening and the closing
characters or HTML elements. In consequence, the use of openings “{“
and closings “}” is mandatory, even for single line instructions:
cshtml// This won’t work in Razor. Content has to be wrapped between { } if( i < 1 ) int myVar=0;
vbhtml// This won’t work in Razor. Content has to be wrapped between { } If i < 1 Then Dim myVar As int =0 End if
ASP.NET MVC 3 comes with a new view engine named Razor that offers the following benefits:
- Razor syntax is clean and concise, requiring a minimum number of keystrokes.
- Razor is easy to learn, in part because it's based on existing languages like C# and Visual Basic.
- Visual Studio includes IntelliSense and code colorization for Razor syntax.
- Razor views can be unit tested without requiring that you run the application or launch a web server.
@model
syntax for specifying the type being passed to the view.@* *@
comment syntax.- The ability to specify defaults (such as
layoutpage
) once for an entire site. - The
Html.Raw
method for displaying text without HTML-encoding it. - Support for sharing code among multiple views (_viewstart.cshtml or _viewstart.vbhtml files).
Partial View
A partial view is like as user control in Asp.Net Web forms that is used for code re-usability. Partial views helps us to reduce code duplication. Hence partial views are reusable views like as Header and Footer views.We can use partial view to display blog comments, product category, social bookmarks buttons, a dynamic ticker, calendar etc. For understanding the different rendering ways of partial view refer the article RenderPartial vs RenderAction vs Partial vs Action in MVC Razor
Creating A Partial ViewA partial view has same file extension(.cshtml) as regular view. To create a partial view do right click on shared folder (\Views\Shared) in solution explorer and click on "Add New View" option and then give the name for partial viewRendering Partial ViewA partial view is rendered by using the ViewUserControl class that is inherited/derived from the ASP.NET UserControl class. The Partial, RenderPartial, RenderAction helper methods are used to render partial view in mvc3 razor.
The main difference between above two methods is that the Partial helper method renders a partial view into a string while RenderPartial method writes directly into the response stream instead of returning a string.- <div> @Html.Partial("_Comments") </div>
- <div> @{Html.RenderPartial("_Comments");} </div>
- <div> @{Html.RenderAction("_Category","Home");} </div>
i.e.
- Partial
or RenderPartial methods are used when a model for the page is already
populated with all the information. For example in a blog to show an
article comment we would like to use Partial or RenderPartial methods
since an article information are already populated in the model.
- RenderAction
method is used when some information is need to show on multiple pages.
Hence partial view should have its own model. For example to category
list of articles on each and every page we would like to use
RenderAction method since the list of category is populated by
different model.
Render Partial View Using jQuerySometimes we need to load a partial view with in a popup on run time like as login box, then we can use jQuery to make an AJAX request and render a Partial View into the popup. In order to load a partial view with in a div we need to do like as:
- <script type="text/jscript">
- $('#divpopup').load('/shared/_ProductCategory’);
- </script>
Razor Basics
MVC 3 ships with a new view-engine option called “Razor”
(in additionto continuing to support/enhance the existing .aspx view engine). You can learn more about Razor, why we are introducing it, and the syntax it supports from my Introducing Razor blog post. If you haven’t read that post yet, take a few minutes and read it now (since the rest of this post will assume you have read it). Once you’ve read the Introducing Razor post, also read my ASP.NET MVC 3 Preview post and look over the ASP.NET MVC 3 Razor sample I included in it.
What are Layouts?
typically want to maintain a consistent look and feel across all of the pages within your web-site/application. ASP.NET 2.0 introduced the concept of “master pages” which helps enable this when using .aspx based pages or templates. Razor also supports this concept with a feature called “layouts” – which allow you to define a common site template, and then inherit its look and feel across all the views/pages on your site.
Difference between 3-tier architecture and MVC?At first glance, the three tiers may seem similar to the MVC (Model View Controller) concept; however, topologically they are different. A fundamental rule in a three-tier architecture is the client tier never communicates directly with the data tier; in a three-tier model all communication must pass through the middleware tier. Conceptually the three-tier architecture is linear. However, the MVC architecture is triangular: the View sends updates to the Controller, the Controller updates the Model, and the View gets updated directly from the Model.
Persisting Data with TempData
TempData is used to pass data from current request to subsequent request (means redirecting from one page to another). It’s life is very short and lies only till the target view is fully loaded. But you can persist data in TempData by calling Keep() method.return View() and return RedirectToAction()
return Redirect() and return RedirectToRoute()
1. Return View doesn't make a new requests, it just renders the view without changing URLs in the browser's address bar.2. Return RedirectToAction makes a new requests and URL in the browser's address bar is updated with the generated URL by MVC.3. Return Redirect also makes a new requests and URL in the browser's address bar is updated, but you have to specify the full URL to redirect4. Between RedirectToAction and Redirect, best practice is to use RedirectToAction for anything dealing with your application actions/controllers. If you use Redirect and provide the URL, you'll need to modify those URLs manually when you change the route table.5. RedirectToRoute redirects to a specific route defined in the Route table.Difference between Routing and URL RewritingMany developers compare routing to URL rewriting that is wrong. Since both the approaches are very much different. Moreover, both the approaches can be used to make SEO friendly URLs. Below is the main difference between these two approaches.- URL rewriting is focused on mapping one URL (new url) to another URL (old url) while routing is focused on mapping a URL to a resource.
- Actually, URL rewriting rewrites your old url to new one while routing never rewrite your old url to new one but it map to the original route.
Html.RenderPartial- This method result will be directly written to the HTTP response stream means it used the same TextWriter object as used in the current webpage/template.
- This method returns void.
- Simple to use and no need to create any action.
- RenderPartial method is useful used when the displaying data in the partial view is already in the corresponding view model.For example : In a blog to show comments of an article, we would like to use RenderPartial method since an article information with comments are already populated in the view model.
1. @{Html.RenderPartial("_Comments");}- This method is faster than Partial method since its result is directly written to the response stream which makes it fast.
Html.RenderAction- This method result will be directly written to the HTTP response stream means it used the same TextWriter object as used in the current webpage/template.
- For this method, we need to create a child action for the rendering the partial view.
- RenderAction method is useful when the displaying data in the partial view is independent from corresponding view model.For example : In a blog to show category list on each and every page, we would like to use RenderAction method since the list of category is populated by the different model.
1. @{Html.RenderAction("Category","Home");}- This method is the best choice when you want to cache a partial view.
- This method is faster than Action method since its result is directly written to the HTTP response stream which makes it fast.
Html.Partial- Renders the partial view as an HTML-encoded string.
- This method result can be stored in a variable, since it returns string type value.
- Simple to use and no need to create any action.
- Partial method is useful used when the displaying data in the partial view is already in the corresponding view model.For example : In a blog to show comments of an article, we would like to use RenderPartial method since an article information with comments are already populated in the view model.
1. @Html.Partial("_Comments")Html.Action- Renders the partial view as an HtmlString .
- For this method, we need to create a child action for the rendering the partial view.
- This method result can be stored in a variable, since it returns string type value.
- Action method is useful when the displaying data in the partial view is independent from corresponding view model.For example : In a blog to show category list on each and every page, we would like to use Action method since the list of category is populated by the different model.
1. @{Html.Action("Category","Home");}- This method is also the best choice when you want to cache a partial view.
Bundling and minification in MVC3 and Asp.Net 4.0
Posted By : Shailendra Chauhan, 05 Jan 2013Updated On : 05 Jan 2013Version Support : MVC3 & Asp.Net 4.0Keywords : bundling and minification in .net framework 4.0, bundling minification in visual studio 2010,bundling minification in asp.net 4.0 and asp.net mvc3You can also implement bundling and minification techniques with in Asp.net MVC3 and Asp.net 4.0. In previous article Asp.net MVC 4 performance optimization with bundling and minification, I have explained both the techniques, Now I would like to share you could you achieve this functionality with .Net Framework 4.0.Bundling and MinificationCreating Bundle
Now create the bundle for your css and js files with in the Global.asax file as shown below.
1. public static void RegisterBundles(BundleCollection bundles)
2. {
3. //Creating bundle for your css files
4. bundles.Add(new StyleBundle("~/Content/css").Include("~/Content/mystyle.min.css",
5. "~/Content/site.min.css"));
6. //Creating bundle for your js files
7. bundles.Add(new ScriptBundle("~/bundles/jquery").Include(
8. "~/Scripts/jquery-1.5.1.min.js",
9. "~/Scripts/jquery.validate.min.js",
10. "~/Scripts/jquery.validate.unobtrusive.min.js"));
11.}
Here, I have created the bundle of all required css and js files. You can also add your own css and js files with complete path using Include method.
Registering Bundle
You need to register above created bundles with in Application_Start event of Global.asax like as
1. protected void Application_Start()
2. {
3. RegisterBundles(BundleTable.Bundles);
4. // Other Code is removed for clarity
5. }
Adding Bundles to Layout Page in MVC3
Now you can add the above created style and script bundles to the Layout page or where you want to use as shown below:
1. @System.Web.Optimization.Styles.Render("~/Content/css")
2. @System.Web.Optimization.Scripts.Render("~/bundles/jquery")
Adding Bundles to Master Page in Asp.Net 4.0
Now you can add the above created style and script bundles to the Master page or where you want to use as shown below:
1. <%: Styles.Render("~/Content/css") %>
2. <%: Scripts.Render("~/bundles/jquery")%>
In Asp.Net 4.0 you also required to add System.Web.Optimization namespace and assembly Microsoft.AspNet.Web.Optimization.WebForms reference to the web.config file of your Asp.Net 4.0 project as shown below:
1. <system.web>
2. <compilation debug="true" targetFramework="4.0" />
3. <pages>
4. <namespaces>
5. <add namespace="System.Web.Optimization" />
6. </namespaces>
7. <controls>
8. <add assembly="Microsoft.AspNet.Web.Optimization.WebForms" namespace="Microsoft.AspNet.Web.Optimization.WebForms" tagPrefix="webopt" />
9. </controls>
10. </pages>
11.<!-- Other Code is removed for clarity -->
12.</sytem.web >
You need not to do any changes in web.config file of your MVC3 project.
Enabling Bundling and Minification in debug mode
Bundling and minification doesn't work in debug mode. So to enable this features you need to add below line of code with in Application_Start event of Global.asax.
1. protected void Application_Start()
2. {
3. BundleConfig.RegisterBundles(BundleTable.Bundles);
4. //Enabling Bundling and Minification
5. BundleTable.EnableOptimizations = true;
6. // Other Code is removed for clarity
7. }
Minification
Minification is technique for removing unnecessary characters (like white space, newline, tab) and comments from the JavaScript and CSS files to reduce the size which cause improved load times of a webpage. There are so many tools for minifying the js and css files. JSMin and YUI Compressor are two most popular tools for minifying the js and css files. Use these tools for minifiying your css and js files and use in your application with ".min" suffix. So that you can easily identified that this is a minimize version of your css or js file.
Donut caching..Donut Caching was introduced which cached only one copy of the entire page for all the user except for a small part which remain dynamic. This small part act like as a hole in the cached content and much like a donut.Donut caching is very useful in the scenarios where most of the elements in your page are rarely changed except the few sections that dynamically change, or changed based on a request parameter.Donut Hole CachingDonut Hole Caching is the inverse of Donut caching means while caching the entire page itDonut Hole caching is very useful in the scenarios where most of the elements in your page are dynamic except the few sections that rarely change, or changed based on a request parameter. Asp.Net MVC has great support for Donut Hole caching through the use of Child Actions.1. [ChildActionOnly]2. [OutputCache(Duration=60)]3. public ActionResult CategoriesList()4. {5. // Get categories list from the database and6. // pass it to the child view7. ViewBag.Categories = Model.GetCategories();8. return View();9. }View with Donut Hole CachingNow call the above action method "CategoriesList" from the parent view as shown below:1. <h1>MVC Donut Hole Caching Demo</h1>2.3. <div>@Html.Action("CategoriesList")</div>4.5. <!-- Rest of the page with non cacheable content goeSecuring Asp.Net MVC Application by using Authorize Attribute
Authorization is the process of determining the rights of an authenticated user for accessing the application's resources. The Asp.Net MVC Framework has a AuthorizeAttribute filter for filtering the authorized user to access a resource. Refer this article for Custom Authentication and Authorization in ASP.NET MVCAuthorize Attribute PropertiesPropertiesDescriptionRolesGets or sets the roles required to access the controller or action method.UsersGets or sets the user names required to access the controller or action method.Filtering Users by Users Property
Suppose you want to allow the access of AdminProfile to only shailendra and mohan users then you can specify the authorize users list to Users property as shown below.
1. [Authorize(Users = "shailendra,mohan")]
2. public ActionResult AdminProfile()
3. {
4. return View();
5. }
Filtering Users by Roles Property
Suppose you want to allow the access of AdminProfile action to only Admin and SubAdmin roles then you can specify the authorize roles list to Users property as shown below.
1. [Authorize(Roles = "Admin,SubAdmin")]
2. public ActionResult AdminProfile()
3. {
4. return View();
5. }
MVC Data Annotations for Model Validation
Posted By : Shailendra Chauhan, 27 Jul 2012Updated On : 31 Jan 2013Keywords : validation with the data annotation validators,validate model data using dataannotations attributes,asp.net mvc 3 viewmodel data annotation,mvc dataannotation error messagesData validation is a key aspect for developing web application. In Asp.net MVC, we can easily apply validation to web application by using Data Annotation attribute classes to model class. Data Annotation attribute classes are present in System.ComponentModel.DataAnnotations namespace and are availlable to Asp.net projects like Asp.net web application & website, Asp.net MVC, Web forms and also to Entity framework orm models.Data Annotations help us to define the rules to the model classes or properties for data validation and displaying suitable messages to end users.
Data Annotation Validator Attributes
1. DataType
Specify the datatype of a property2. DisplayName
specify the display name for a property.3. DisplayFormat
specify the display format for a property like different format for Date proerty.4. Required
Specify a property as required.5. ReqularExpression
validate the value of a property by specified regular expression pattern.6. Range
validate the value of a property with in a specified range of values.7. StringLength
specify min and max length for a string property.8. MaxLength
specify max length for a string property.9. Bind
specify fields to include or exclude when adding parameter or form values to model properties.10. ScaffoldColumn
specify fields for hiding from editor forms.Designing the model with Data Annotations
1.
2. using System.ComponentModel;
3. using System.ComponentModel.DataAnnotations;
4. using System.Web.Mvc;
5. namespace Employee.Models
6. {
7. [Bind(Exclude = "EmpId")]
8. public class Employee
9. {
10. [ScaffoldColumn(false)]
11. public int EmpId { get; set; }
12.
13. [DisplayName("Employee Name")]
14. [Required(ErrorMessage = "Employee Name is required")]
15. [StringLength(100,MinimumLength=3)]
16. public String EmpName { get; set; }
17.
18. [Required(ErrorMessage = "Employee Address is required")]
19. [StringLength(300)]
20. public string Address { get; set; }
21.
22. [Required(ErrorMessage = "Salary is required")]
23. [Range(3000, 10000000,ErrorMessage = "Salary must be between 3000 and 10000000")]
24. public int Salary{ get; set; }
25.
26. [Required(ErrorMessage = "Please enter your email address")]
27. [DataType(DataType.EmailAddress)]
28. [Display(Name = "Email address")]
29. [MaxLength(50)]
30. [RegularExpression(@"[a-z0-9._%+-]+@[a-z0-9.-]+\.[a-z]{2,4}", ErrorMessage = "Please enter correct email")]
31. public string Email { get; set; }
32. }
33.}
Once we have define validation to the model by using data annotations, these are automatically used by Html Helpers in views. For client side validation to work, please ensure that below two <SCRIPT> tag references are in the view.
1. <script src="@Url.Content("~/Scripts/jquery.validate.min.js")" type="text/javascript"></script>
2. <script src="@Url.Content("~/Scripts/jquery.validate.unobtrusive.min.js")" type="text/javascript"></script>
Presenting the model in the view
1. @model Employee.Models
2. @{
3. ViewBag.Title = "Employee Details";
4. Layout = "~/Views/Shared/_Layout.cshtml";
5. }
6. @using (Html.BeginForm())
7. {
8. <div class="editor-label">
9. @Html.LabelFor(m => m.EmpName)
10. </div>
11. <div class="editor-field">
12. @Html.TextBoxFor(m => m.EmpName)
13. @Html.ValidationMessageFor(m => m.EmpName)
14. </div>
15. <div class="editor-label">
16. @Html.LabelFor(m => m.Address)
17. </div>
18. <div class="editor-field">
19. @Html.TextBoxFor(m => m.Address)
20. @Html.ValidationMessageFor(m => m.Address)
21. </div>
22. <div class="editor-label">
23. @Html.LabelFor(m => m.Salary)
24. </div>
25. <div class="editor-field">
26. @Html.TextBoxFor(m => m.Salary)
27. @Html.ValidationMessageFor(m => m.Salary)
28. </div>
29. <div class="editor-label">
30. @Html.LabelFor(m => m.Email)
31. </div>
32. <div class="editor-field">
33. @Html.TextBoxFor(m => m.Email)
34. @Html.ValidationMessageFor(m => m.Email)
35. </div>
36. <p> <input type="submit" value="Save" />
37. </p>
38.}
ViewModel in ASP.NET MVC
In ASP.NET MVC, ViewModel is a class that contains the fields which are represented in the strongly-typed view. It is used to pass data from controller to strongly-typed view.Key Points about ViewModel
1. ViewModel contain fields that are represented in the view (for LabelFor,EditorFor,DisplayFor helpers)2. ViewModel can have specific validation rules using data annotations or IDataErrorInfo.3. ViewModel can have multiple entities or objects from different data models or data source.ViewModel Example
Designing ViewModel
1. public class UserLoginViewModel
2. {
3. [Required(ErrorMessage = "Please enter your username")]
4. [Display(Name = "User Name")]
5. [MaxLength(50)]
6. public string UserName { get; set; }
7. [Required(ErrorMessage = "Please enter your password")]
8. [Display(Name = "Password")]
9. [MaxLength(50)]
10. public string Password { get; set; }
11.}
Presenting the viewmodel in the view
1. @model MyModels.UserLoginViewModel
2. @{
3. ViewBag.Title = "User Login";
4. Layout = "~/Views/Shared/_Layout.cshtml";
5. }
6. @using (Html.BeginForm())
7. {
8. <div class="editor-label">
9. @Html.LabelFor(m => m.UserName)
10.</div>
11.<div class="editor-field">
12. @Html.TextBoxFor(m => m.UserName)
13. @Html.ValidationMessageFor(m => m.UserName)
14.</div>
15.<div class="editor-label">
16. @Html.LabelFor(m => m.Password)
17.</div>
18.<div class="editor-field">
19. @Html.PasswordFor(m => m.Password)
20. @Html.ValidationMessageFor(m => m.Password)
21.</div>
22.<p>
23. <input type="submit" value="Log In" />
24.</p>
25.</div>
26.}
Working with Action
1. public ActionResult Login()
2. {
3. return View();
4. }
5. [HttpPost]
6. public ActionResult Login(UserLoginViewModel user)
7. {
8. // To acces data using LINQ
9. DataClassesDataContext mobjentity = new DataClassesDataContext();
10. if (ModelState.IsValid)
11.{
12.try
13. {
14. var q = mobjentity.tblUsers.Where(m => m.UserName == user.UserName && m.Password == user.Password).ToList();
15. if (q.Count > 0)
16. {
17. return RedirectToAction("MyAccount");
18. }
19. else
20. {
21. ModelState.AddModelError("", "The user name or password provided is incorrect.");
22. }
23. }
24. catch (Exception ex)
25. {
26. }
27. }
28. return View(user);
29.}
ViewModel
1. In ViewModel put only those fields/data that you want to display on the view/page.2. Since view reperesents the properties of the ViewModel, hence it is easy for rendering and maintenance.3. Use a mapper when ViewModel become more complex.In this way, ViewModel help us to organize and manage data in a strongly-typed view with more flexible way than complex objects like models or ViewBag/ViewData objects.
ViewData vs ViewBag vs TempData vs Session
In ASP.NET MVC there are three ways - ViewData, ViewBag and TempData to pass data from controller to view and in next request. Like WebForm, you can also use Session to persist data during a user session. Now question is that when to use ViewData, VieBag, TempData and Session. Each of them has its own importance. In this article, I am trying to explain the differences among these four.ViewData
1. ViewData is a dictionary object that is derived from ViewDataDictionary class.2. ViewData is used to pass data from controller to corresponding view.3. It’s life lies only during the current request.4. If redirection occurs then it’s value becomes null.5. It’s required typecasting for getting data and check for null values to avoid error.ViewBag
1. ViewBag is a dynamic property that takes advantage of the new dynamic features in C# 4.0.2. Basically it is a wrapper around the ViewData and also used to pass data from controller to corresponding view.3. It’s life also lies only during the current request.4. If redirection occurs then it’s value becomes null.5. It doesn’t required typecasting for getting data.TempData
1. TempData is a dictionary object that is derived from TempDataDictionary class and stored in short lives session.2. TempData is used to pass data from current request to subsequent request (means redirecting from one page to another).3. It’s life is very short and lies only till the target view is fully loaded.4. It’s required typecasting for getting data and check for null values to avoid error.5. It is used to store only one time messages like error messages, validation messages.Session
1. Session is also used to pass data within the ASP.NET MVC application and Unlike TempData, it never expires.2. Session is valid for all requests, not for a single redirect.3. It’s also required typecasting for getting data and check for null values to avoid error.Difference between Asp.Net MVC and Web FormsAsp.Net Web FormsAsp.Net MVCAsp.Net Web Form follow a traditional event driven development model.Asp.Net MVC is a lightweight and follow MVC (Model, View, Controller) pattern based development model.Asp.Net Web Form has server controls.Asp.Net MVC has html helpers.Asp.Net Web Form has state management (like as view state, session) techniques.Asp.Net MVC has no automatic state management techniques.Asp.Net Web Form has file-based URLs means file name exist in the URLs must have its physically existence.Asp.Net MVC has route-based URLs means URLs are divided into controllers and actions and moreover it is based on controller not on physical file.Asp.Net Web Form follows Web Forms SyntaxAsp.Net MVC follow customizable syntax (Razor as default)In Asp.Net Web Form, Web Forms(ASPX) i.e. views are tightly coupled to Code behind(ASPX.CS) i.e. logic.In Asp.Net MVC, Views and logic are kept separately.Asp.Net Web Form has Master Pages for consistent look and feels.Asp.Net MVC has Layouts for consistent look and feels.Asp.Net Web Form has User Controls for code re-usability.Asp.Net MVC has Partial Views for code re-usability.Asp.Net Web Form has built-in data controls and best for rapid development with powerful data access.Asp.Net MVC is lightweight, provide full control over markup and support many features that allow fast & agile development. Hence it is best for developing interactive web application with latest web standards.Asp.Net Web Form is not Open Source.Asp.Net Web MVC is an Open Source.Setting default submit button in MVC3 razor using jQuery
In Asp.net MVC, sometimes we required to post the form on Enter key press. Asp.net MVC has no default button property like Asp.net. However, we can achieve this functionality by using jQuery in MVC.Set Form DefaultButton Property using jQuery
1. <script type="text/javascript"> $(document).ready(function (){ $("#MyForm").keypress(function (e) { kCode = e.keyCode || e.charCode //for cross browser
2. if (kCode == 13) { var defaultbtn = $(this).attr("DefaultButton");
3. $("#" + defaultbtn).click();
4. return false;
5. }
6. });
7. });
8. </script>
9. @using (Html.BeginForm("Index", "Home", FormMethod.Post, new { DefaultButton = "SubmitButton", id = "MyForm" }))
10.{
11. @Html.TextBox("txtname")
12. <span>Please Enter value and then press Enter Key</span><br />
13. <input type="submit" name="btnSubmit" id="SubmitButton" value="Submit" />
14.}
Handling multiple submit buttons on the same form - MVC Razor
Posted By : Shailendra Chauhan, 16 Nov 2012Updated On : 31 Jan 2013Version Support : MVC3 & MVC4Keywords : how to use more than one submit button on the same page in mvc razor, handle submit button with cancel button in mvc razor, handle cancel button click in mvc razorSometimes you required to have more than one submit buttons on the same form in mvc razor. In that case, how will you handle the click event of different buttons on your form?In this article, I am going to expose the various ways for handling multiple buttons on the same form. Suppose you have a user signup form like as below:
Method 1 - Submit the form for each button
In this way each submit button will post the form to server but provides the different values - Save, Submit and NULL respectively for the commands. On the basis of command name we can implement our own logic in the controller's action method.
MultipleCommand.cshtml
1. @using (Html.BeginForm("MultipleCommand", "Home", FormMethod.Post, new { id = "submitForm" }))
2. {
3. <fieldset>
4. <legend>Registration Form</legend>
5. <ol>
6. <li>
7. @Html.LabelFor(m => m.Name)
8. @Html.TextBoxFor(m => m.Name, new { maxlength = 50 })
9. @Html.ValidationMessageFor(m => m.Name)
10. </li>
11. <li>
12. @Html.LabelFor(m => m.Address)
13. @Html.TextAreaFor(m => m.Address, new { maxlength = 200 })
14. @Html.ValidationMessageFor(m => m.Address)
15. </li>
16. <li>
17. @Html.LabelFor(m => m.MobileNo)
18. @Html.TextBoxFor(m => m.MobileNo, new { maxlength = 10 })
19. @Html.ValidationMessageFor(m => m.MobileNo)
20. </li>
21. </ol>
22. <button type="submit" id="btnSave" name="Command" value="Save">Save</button>
23. <button type="submit" id="btnSubmit" name="Command" value="Submit">Submit</button>
24. <button type="submit" id="btnCancel" name="Command" value="Cancel" onclick="$('#submitForm').submit()">Cancel (Server Side)</button>
25. </fieldset>
26.}
Method 2 - Introducing Second From
We can also introduce the second form for handling Cancel button click. Now, on Cancel button click we will post the second form and will redirect to the home page.
MultipleCommand.cshtml
1. @using (Html.BeginForm("MultipleCommand", "Home", FormMethod.Post, new { id = "submitForm" }))
2. {
3. <fieldset>
4. <legend>Registration Form</legend>
5. <ol>
6. <li>
7. @Html.LabelFor(m => m.Name)
8. @Html.TextBoxFor(m => m.Name, new { maxlength = 50 })
9. @Html.ValidationMessageFor(m => m.Name)
10. </li>
11. <li>
12. @Html.LabelFor(m => m.Address)
13. @Html.TextAreaFor(m => m.Address, new { maxlength = 200 })
14. @Html.ValidationMessageFor(m => m.Address)
15. </li>
16. <li>
17. @Html.LabelFor(m => m.MobileNo)
18. @Html.TextBoxFor(m => m.MobileNo, new { maxlength = 10 })
19. @Html.ValidationMessageFor(m => m.MobileNo)
20. </li>
21. </ol>
22. <button type="submit" id="btnSave" name="Command" value="Save">Save</button>
23. <button type="submit" id="btnSubmit" name="Command" value="Submit">Submit</button>
24. <button type="submit" id="btnCancelSecForm" name="Command" value="Cancel" onclick="$('#cancelForm').submit()"> Cancel (Server Side by Second Form)</button>
25. </fieldset>
26.}
27.@using (Html.BeginForm("MultipleButtonCancel", "Home", FormMethod.Post, new { id = "cancelForm" })) { }
Method 3 - Introducing Client Side Script
We can also use javascript or jquery for handling Cancel button click. Now, on Cancel button click we will directly redirect to the home page. In this way, there is no server side post back and this is the more convenient way to handle the cancel button click.
MultipleCommand.cshtml
1. <button type="submit" id="btnSave" name="Command" value="Save">Save</button>
2. <button type="submit" id="btnSubmit" name="Command" value="Submit">Submit</button>
3. <button name="ClientCancel" type="button" onclick=" document.location.href = $('#cancelUrl').attr('href');">Cancel (Client Side)</button>
4. <a id="cancelUrl" href="@Html.AttributeEncode(Url.Action("Index", "Home"))" style="display:none;"></a>
WebGrid with Insert Update and Delete Operations
Many developers want to do Insert, Update and Delete with in WebGrid like as GridView, but don't know how to do it. This article will help you to do the CRUD operations with in WebGrid.
How to Enable and Disable Client-Side Validation in MVC
Posted By : Shailendra Chauhan, 15 Dec 2012Updated On : 15 Dec 2012Version Support : MVC3 & MVC4Keywords : enabling and disabling client side model validation in mvc3 razor,enable and disable client side validation for a view in mvc3 or MVC4 razorMVC3 & MVC4 supports unobtrusive client-side validation. In which validation rules are defined using attributes added to the generated HTML elements. These rules are interpreted by the included JavaScript library and uses the attribute values to configure the jQuery Validation library which does the actual validation work. In this article, I would like to demonstrate various ways for enabling or disabling the client side validation.Enable Client-Side Validation in MVC
For enabling client side validation, we required to include the jQuery min, validate & unobtrusive scripts in our view or layout page in the following order.
1. <script src="@Url.Content("~/Scripts/jquery-1.6.1.min.js")" type="text/javascript"></script>
2. <script src="@Url.Content("~/Scripts/jquery.validate.js")" type="text/javascript"></script>
3. <script src="@Url.Content("~/Scripts/jquery.validate.unobtrusive.js")" type="text/javascript"></script>
The order of included files as shown above, is fixed since below javascript library depends on top javascript library.
Enabling and Disabling Client-Side Validation at Application Level
We can enable and disable the client-side validation by setting the values of ClientValidationEnabled & UnobtrusiveJavaScriptEnabled keys true or false. This setting will be applied to application level.
1. <appSettings>
2. <add key="ClientValidationEnabled" value="true"/>
3. <add key="UnobtrusiveJavaScriptEnabled" value="true"/>
4. </appSettings>
For client-side validation, the values of above both the keys must be true. When we create new project using Visual Studio in MVC3 or MVC4, by default the values of both the keys are set to true.
We can also enable the client-side validation programmatically. For this we need to do code with in the Application_Start() event of the Global.asax, as shown below.
1. protected void Application_Start()
2. {
3. //Enable or Disable Client Side Validation at Application Level
4. HtmlHelper.ClientValidationEnabled = true;
5. HtmlHelper.UnobtrusiveJavaScriptEnabled = true;
6. }
Enabling and Disabling Client-Side Validation for Specific View
We can also enable or disable client-side validation for a specific view. For this we required to enable or disable client side validation inside a Razor code block as shown below. This option will overrides the application level settings for that specific view.
1. @model MvcApp.Models.Appointment
2. @{
3. ViewBag.Title = "Make A Booking";
4. HtmlHelper.ClientValidationEnabled = false;
5. }
6. ...
Difference between WCF and Web API and WCF REST and Web Service
The .Net framework has a number of technologies that allow you to create HTTP services such as Web Service, WCF and now Web API. There are a lot of articles over the internet which may describe to whom you should use. Now a days, you have a lot of choices to build HTTP services on .NET framework. In this article, I would like to share my opinion with you over Web Service, WCF and now Web API. For more information about Web API refers What is Web API and why to use it ?.Web Service
1. It is based on SOAP and return data in XML form.2. It support only HTTP protocol.3. It is not open source but can be consumed by any client that understands xml.4. It can be hosted only on IIS.WCF
1. It is also based on SOAP and return data in XML form.2. It is the evolution of the web service(ASMX) and support various protocols like TCP, HTTP, HTTPS, Named Pipes, MSMQ.3. The main issue with WCF is, its tedious and extensive configuration.4. It is not open source but can be consumed by any client that understands xml.5. It can be hosted with in the applicaion or on IIS or using window service.WCF Rest
1. To use WCF as WCF Rest service you have to enable webHttpBindings.2. It support HTTP GET and POST verbs by [WebGet] and [WebInvoke] attributes respectively.3. To enable other HTTP verbs you have to do some configuration in IIS to accept request of that particular verb on .svc files4. Passing data through parameters using a WebGet needs configuration. The UriTemplate must be specified5. It support XML, JSON and ATOM data format.Web API
1. This is the new framework for building HTTP services with easy and simple way.2. Web API is open source an ideal platform for building REST-ful services over the .NET Framework.3. Unlike WCF Rest service, it use the full featues of HTTP (like URIs, request/response headers, caching, versioning, various content formats)4. It also supports the MVC features such as routing, controllers, action results, filter, model binders, IOC container or dependency injection, unit testing that makes it more simple and robust.5. It can be hosted with in the application or on IIS.6. It is light weight architecture and good for devices which have limited bandwidth like smart phones.7. Responses are formatted by Web API’s MediaTypeFormatter into JSON, XML or whatever format you want to add as a MediaTypeFormatter.choose between WCF or WEB API
1. Choose WCF when you want to create a service that should support special scenarios such as one way messaging, message queues, duplex communication etc.2. Choose WCF when you want to create a service that can use fast transport channels when available, such as TCP, Named Pipes, or maybe even UDP (in WCF 4.5), and you also want to support HTTP when all other transport channels are unavailable.3. Choose Web API when you want to create a resource-oriented services over HTTP that can use the full features of HTTP (like URIs, request/response headers, caching, versioning, various content formats).4. Choose Web API when you want to expose your service to a broad range of clients including browsers, mobiles, iphone and tablets.Difference Between Generalization and Specialization
Keywords : generalization and specialization with example, understanding generalization and specializationThe process of extracting common characteristics from two or more classes and combining them into a generalized superclass, is called Generalization. The common characteristics can be attributes or methods. Generalization is represented by a triangle followed by a line.
Let’s take an example of Bank Account; A Bank Account is of two types – Current Account and Saving Account. Current Account and Saving Account inherits the common/ generalized properties like Account Number, Account Balance etc. from a Bank Account and also have their own specialized properties like interest rate etc.
Understanding Var and IEnumerable with LINQ
In previous article I explain the difference between IEnumerable and IQuerable, IEnumerable and IList. In this article I would like to share my opinion on Var and IEnumerable with LINQ. IEnumerable is an interface that can move forward only over a collection, it can’t move backward and between the items. Var is used to declare implicitly typed local variable means it tells the compiler to figure out the type of the variable at compilation time. A var variable must be initialized at the time of declaration. Both have its own importance to query data and data manipulation.Var Type with LINQ
Since Var is anonymous types, hence use it whenever you don't know the type of output or it is anonymous. In LINQ, suppose you are joining two tables and retrieving data from both the tables then the result will be an Anonymous type.
1. var q =(from e in tblEmployee
2. join d in tblDept on e.DeptID equals d.DeptID
3. select new
4. {
5. e.EmpID,
6. e.FirstName,
7. d.DeptName,
8. });
In above query, result is coming from both the tables so use Var type.
1. var q =(from e in tblEmployee where e.City=="Delhi" select new {
2. e.EmpID,
3. FullName=e.FirstName+" "+e.LastName,
4. e.Salary
5. });
In above query, result is coming only from single table but we are combining the employee's FirstName and LastName to new type FullName that is annonymous type so use Var type. Hence use Var type when you want to make a "custom" type on the fly.
More over Var acts like as IQueryable since it execute select query on server side with all filters. Refer below examples for explanation.
IEnumerable Example
1. MyDataContext dc = new MyDataContext ();
2. IEnumerable<Employee> list = dc.Employees.Where(p => p.Name.StartsWith("S"));
3. list = list.Take<Employee>(10);
Generated SQL statements of above query will be :
1. SELECT [t0].[EmpID], [t0].[EmpName], [t0].[Salary] FROM [Employee] AS [t0] WHERE [t0].[EmpName] LIKE @p0
Notice that in this query "top 10" is missing since IEnumerable filters records on client side
Var Example
1. MyDataContext dc = new MyDataContext ();
2. var list = dc.Employees.Where(p => p.Name.StartsWith("S"));
3. list = list.Take<Employee>(10);
WPF stands for Windows Presentation Foundation. It is an application programming Interface for developing rich UI on Windows. WPF is introduced in .NET 3.0. By the use of WPF we can create two and three dimensional graphics, animations etc.What is XAML and how it is related to WPF?XAML is a new mark up language which is used for defining UI elements and its relationships with other UI elements. The XAML is introduced by WPF in .NET 3.0 WPF uses XAML for UI design.Does XAML file compiled or Parsed?By default XAML files are compiled, But we do have options to let it be parsed.What is the root namespace used for Animations and 3D rendering in WPF?System.Windows.Media namespace.What are the names of main assemblies used by WPF?a)WindowsBaseb)PresentationCorec)PresentationFoundationDescribe the types of documents supported by WPF?There are two kinds of document supported by WPFa)Flow format:Flow format document adjusts as per screen size and resolutionb)Fixed Format:Fixed Format document does not adjust as per screen size and resolutionWhat namespaces are needed to host a WPF control in Windows form application?The following namespaces needs to be referenced:a)PresentationCore.dllb)PresentationFramework.dllc)UIAutomationProvider.dlld)UIAutomationTypes.dlle)WindowsBase.dllWhat is dependency property?A property that is support by the WPF property system is known as a dependency property.Example:<StackPanel Canvas.Top="50" Canvas.Left="50" DataContext="{Binding Source={StaticResource XmlTeamsSource}}"><Button Height=”30” width=”80” Content="{Binding XPath=Team/@TeamName}" Background="Red" Style="{StaticResource GreenButtonStyle}"/></StackPanel>Here Height, width, Baground etc are regular property but DataContext, Style, ‘Canves.Lef’t, ‘Canves .right ‘are the dependency on this example, that is call dependency property. I thing, all WPF binding control are dependency property.What is routed event in wpf?A routed event is a CLR event that is support by an instance of the Routed Event class and is processed by the Windows Presentation Foundation (WPF) event system.For example: Event bubbling is good concept routed event.<StackPanel Background="LightGray" Orientation="Horizontal" Button.Click="CommonClickHandler"><Button Name="YesButton" Width="Auto" >Yes</Button></StackPanel>What does mean "x:" prefix in xaml code?It is an xml namespace that usually refers to the xaml namespace.Example:xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"What is XBAP Stands?XBAP Stands for XAML Browser application.What is a .baml file?An XAML file is compiled in .net, become a file with a .baml extension, which stand for binary XAML.Types of window in wpf?Normal windowNavigate windowPage WindowWhat is dispatcher Object?It is the main of all WPF controls which Take care UI Thread.What are the method in DependencyObject in WPF?ClearValueSetValueGetValueWhat types of control in wpf?Content controlLayout controlItem controlCan you create Window service in WPF?No! , It use for UI, we create a cervices using c# or other language.What is XBAP?XBAP is stand for XAML Browser Application which is a new Windows technology used for creating Rich Internet Applications.While windows applications are normally compiled to an .exe file, browser applications are compiled to an extension .xbap and can be run inside Internet Explorer.Xbap applications are run within a security sandbox to prevent untrusted applications from controlling local system resources. (e.gdeleting local files)What is the differenece between System.Windows.dll and System.Windows.Browser.dll?1)System.Windows.dll:-This Assembly includes many of the classes for building Silverlight user interfaces,including basic elements,Shapes and Brushes,classes that support animation and databinding,and the version of the FileOpenDialog that works with isolated storage.2)System.Windows.Browser.dll:-This Assembly contains classes for interacting with HTML Elements.Name the Classes that contains arbitrary content in WPF?1)ContentControl:- A single arbitrary object.2)HeaderedContentControl:- A header and a single item, both of which are arbitrary objects.3)ItemsControl:- A collection of arbitrary objects.4)HeaderedItemsControl:- A header and a collection of items, all of which are arbitrary objects.Name the Events that are implemented by NavigationService?1)Navigating:- Occurs when a new navigation is requested. Can be used to cancel the navigation.2)NavigationProgress:- Occurs periodically during a download to provide navigation progress information.3)Navigated:- Occurs when the page has been located and downloaded.4)NavigationStopped:- Occurs when the navigation is stopped (by calling StopLoading), or when a new navigation is requested while a current navigation is in progress.5)NavigationFailed:- Occurs when an error is raised while navigating to the requested content.6)LoadCompleted:- Occurs when content that was navigated to is loaded and parsed, and has begun rendering.7)FragmentNavigation:- Occurs when navigation to a content fragment begins, which happens:a)Immediately, if the desired fragment is in the current content.b)After the source content has been loaded, if the desired fragment is in different content.What is the default Orientation of Stack Panel?The Default Orientation of Stack Panel is 'Vertical'.What are the major subsystems of the Windows Presentation Foundation?1) Object2) Threading.DispatcherObject3) Windows.DependancyObject4) Windows.Media.Visuals5) Windows.UIElements6) Windows.FrameworkElement7) Windows.Controls.ControlIn Which NameSpace you will find a 'Popup' and 'Thumb' control in WPF?In 'system.windows.controls.primitives' namespaceName Some Methods of MediaElement in WPF?MediaElement has the following methods :-1)Play:-Plays media from the current position.2)Stop:-Stops and resets media to be played from the beginning.3)Pause:-Pauses media at the current position.4)Close:-Closes the media.How to Set Window Title Bar Name at RunTime in WPF?this.Title="TitleName";In Which of the Following Definitions element you will find a 'Width' and 'Height' of a Grid(TAG)1)<Grid.RowDefinitions >2)<Grid.ColumnDefinitions>In <Grid.RowDefinitions > you will find a 'Height'eg: <Grid.RowDefinitions ><RowDefinition Height="50" /></Grid.RowDefinitions>In <Grid.ColumnDefinitions> you will find a 'Width'eg:<Grid.ColumnDefinitions><ColumnDefinition Width="90" /></Grid.ColumnDefinitions>What are the Features of WPF?The Features of WPF are:-1)Device Independent Pixel (DPI).2)Built-In Support for Graphics and Animation.3)Redefine Styles and Control Templates.4)Resource based Approach for every control.5)New Property System & Binding Capabilities.Name the Layout Panels of WPF?These are the five most layout panels of WPF:-1)Grid Panel2)Stack Panel3)Dock Panel4)Wrap Panel5)Canvas PanelWhat are the difference between CustomControls and UserControls in WPF?CustomControl (Extending an existing control):-1)Extends an existing control with additional features.2)Consists of a code file and a default style in Themes/Generic.xaml.3)Can be styled/templated.4)The best approach to build a control library.UserControl (Composition):1)Composes multiple existing controls into a reusable "group".2)Consists of a XAML and a code behind file.3)Cannot be styled/templated.4)Derives from UserControl.What are the Advantages of XAML?1)XAML code is short and clear to read.2)Separation of designer code and logic.3)Graphical design tools like Expression Blend require XAML as source.4)The separation of XAML and UI logic allows it to clearly separate the roles of designer and developer.Which class of the WPF is the base class of all the user-interactive elements?Control class is the base class of all the user-interactive elements in WPF.
What is Markup Extensions?The most common markup extensions used in WPF application programming are Binding, used for data binding expressions, and the resource references StaticResource and DynamicResource.<StackPanel><Border Style="{StaticResource PageBackground}"></Border></StackPanel>What is type convertor?In the section, it was stated that the attribute value must be able to be set by a string. The basic, native handling of how strings are converted into other object types or primitive values is based on the String type itself, in addition to native processing for certain types such as DateTime or Uri. But many WPF types or members of those types extend the basic string attribute processing behavior, in such a way that instances of more complex object types can be specified as strings and attributes.About The X: prefix?The prefix x: was used to map the XAML namespace http://schemas.microsoft.com/winfx/2006/xaml, which is the dedicated XAML namespace that supports XAML language constructs. This x: prefix is used for mapping this XAML namespace in the templates for projects.x:Key: Sets a unique key for each resource in a ResourceDictionary.Example:<object x:Key="stringKeyValue".../>x:Class: Specifies the CLR namespace and class name for the class that provides code-behind for a XAML page.Example:<object x:Class="namespace.classname".../>x:Name: Specifies a run-time object name for the instance that exists in run-time code after an object element is processed.Example:<object x:Name="XAMLNameValue".../>x:Static: Enables a reference that returns a static value that is not otherwise a XAML-compatible property.Example:<object property="{x:Static prefix:typeName.staticMemberName}" .../>x:Type: Constructs a Type reference based on a type name.Example:<object property="{x:Type prefix:typeNameValue}" .../>This example shows you how to create a simple Binding.In this example, you have a Person object with a string property named PersonName. The Person object is defined in the namespace called SDKSample.The following example instantiates the Person object with a PersonName property value of Anil. This is done in the Resources section and assigned an x:Key.XAML<Window xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:ak="clr-namespace:SDKSample"Title="Simple Data Binding Sample"><Window.Resources><ak:Person x:Key="myDataSource" PersonName="Anil"/></Window.Resources><Grid><TextBlock Text="{Binding Path=PersonName, Source={StaticResource myDataSource}}"/></Grid></Window>As a result, the TextBlock appears with the value "Anil".MultiBinding:</TextBlock >
Q.What is the purpose of AccessText?Ans.AccessText enables you to specify displayed text with an embedded keyboard shortcut used to access the corresponding control. Simply precede the desired letter of the text with an underscore.Q.What are the types of Inlines that can be used in a TextBlock?Ans.Span
Bold
Italic
Underline
Hyperlink
Run
LineBreak
InlineUIContainer
Figure
FloaterQ.What is the most common format for declaring a data binding in markup?Ans.Markup extensions are the most common means of declaring a data binding. They are identified by the use of curly brackets ({}).The less common format is XAML.Q.Is xStatic the same as a data binding?Ans.xStatic is another markup extension that is different from the data binding markup extension. It is used to retrieve data from static value members on classes.Q.How many ways are there to deploy WPF applications, and what are they?Ans.There are three ways to deploy WPF applications
as a simple XAML file
an XBAP
or a standard executable.Q.How do navigation applications differ from standard WPF applications?Ans.Navigation applications are based on a web browser metaphor. They use pages instead of windows, and they include a forward and back button by default.Q.What type of elements can be hosted in a ToolBar?Ans.A ToolBar can host any type of .NET class.
Q.What is the purpose of AccessText?Ans.AccessText enables you to specify displayed text with an embedded keyboard shortcut used to access the corresponding control. Simply precede the desired letter of the text with an underscore.Q.What are the types of Inlines that can be used in a TextBlock?Ans.Span
Bold
Italic
Underline
Hyperlink
Run
LineBreak
InlineUIContainer
Figure
FloaterQ.What is the most common format for declaring a data binding in markup?Ans.Markup extensions are the most common means of declaring a data binding. They are identified by the use of curly brackets ({}).The less common format is XAML.Q.Is xStatic the same as a data binding?Ans.xStatic is another markup extension that is different from the data binding markup extension. It is used to retrieve data from static value members on classes.Q.How many ways are there to deploy WPF applications, and what are they?Ans.There are three ways to deploy WPF applications
as a simple XAML file
an XBAP
or a standard executable.Q.How do navigation applications differ from standard WPF applications?Ans.Navigation applications are based on a web browser metaphor. They use pages instead of windows, and they include a forward and back button by default.Q.What type of elements can be hosted in a ToolBar?Ans.A ToolBar can host any type of .NET class.Q.What is the simplest and most common way to componentize a complex UI?Ans.The easiest way to break up a complex UI is to create separate UserControls for related parts and reference them from the original UI.Q.What class is used to save and load the content of a RichTextBox?Ans.To save or load the content of a RichTextBox, use the TextRange class.Q.What are the types of binding in WPF?Ans.There are two types of binding in WPF
Key Binding
Mouse BindingQ.What is the difference between key binding and mouse binding?Ans.KeyBinding allows you to define gestures for commands that consist of a keystroke possibly combined with one or more modifiers, whereas MouseBinding allows you to define gestures for the mouse that can also be optionally combined with modifiers on the keyboard.Q.What type is used to render WPF output to a bitmap?Ans.Use RenderTargetBitmap to render WPF visuals to a bitmap.Q.What is the base class for all renderable types in WPF?Ans.All elements that are renderable must inherit from Visual.Q.What is a repository?Ans.A repository is a type of class that hides the actual data storage mechanism from the rest of the application.Q.What is the purpose of the xName attribute in XAML?Ans.The xName attribute allows you to uniquely identify an instance of an object defined in XAML. The value of the xName attribute can be referenced in the associated C# or VB code.Q.What is a feature of XAML that is available when it is compiled rather than interpreted?Ans.When your XAML is compiled, you can embed procedural code such as C# or VB. For more information about this, look up the markup extension xCode.Q.What is an attached property?Ans.An attached property is a property that is declared by one control and attached to another. It allows the inclusion of additional information with a control for later use by an external source.Q.What kind of documents are supported in WPF?Ans.There are two kind of major document supported in
WPF Fixed format documents
Flow format document.Q.What is the difference between fixed format documents and flow format documents?Ans.Fixed format documents look like PDF format. They display content regardless of screen size and resolution. But Flow format document adjust depending on screen size and resolution.Q.What is the difference between a Panel and a Decorator?Ans.A Panel has a collection of Children that it arranges according to various rules, based on the type of panel.
A Decorator, on the other hand, has only one Child to which it applies some additional set of behavior.Q.What is the feature of silverlight 4?Ans.Silverlight 4 is the current version at the time of writing and it introduced a number of new features, including
Support for Google’s Chrome web browser
Access to the user’s web cam and microphone
Mousewheel input
Enhancements to existing controls, such as the DataGrid
New controls, such as the RichTextBox
Enhanced data binding support
Managed Extensibility Framework support
WCF RIA services
Q.What is the purpose of the xName attribute in XAML?Ans.The xName attribute allows you to uniquely identify an instance of an object defined in XAML. The value of the xName attribute can be referenced in the associated C# or VB code.Q.What is a feature of XAML that is available when it is compiled rather than interpreted?Ans.When your XAML is compiled, you can embed procedural code such as C# or VB. For more information about this, look up the markup extension xCode.Q.What is an attached property?Ans.An attached property is a property that is declared by one control and attached to another. It allows the inclusion of additional information with a control for later use by an external source.Q.What kind of documents are supported in WPF?Ans.There are two kind of major document supported in
WPF Fixed format documents
Flow format document.Q.What is the difference between fixed format documents and flow format documents?Ans.Fixed format documents look like PDF format. They display content regardless of screen size and resolution. But Flow format document adjust depending on screen size and resolution.Q.What is the difference between a Panel and a Decorator?Ans.A Panel has a collection of Children that it arranges according to various rules, based on the type of panel.
A Decorator, on the other hand, has only one Child to which it applies some additional set of behavior.Q.What is the feature of silverlight 4?Ans.Silverlight 4 is the current version at the time of writing and it introduced a number of new features, including
Support for Google’s Chrome web browser
Access to the user’s web cam and microphone
Mousewheel input
Enhancements to existing controls, such as the DataGrid
New controls, such as the RichTextBox
Enhanced data binding support
Managed Extensibility Framework support
WCF RIA servicesQ.What are the types of common file dialogs that WPF supports?Ans.The common file dialogs that WPF supports include SaveFileDialog, OpenFileDialog, and PrintDialog. WPF does not presently have a common dialog for folder selection, font selection, or print preview.Q.What is the name of the FrameworkElement most commonly used to work with media in WPF?Ans.MediaElement is used to play back a variety of audio and video formats.Q.What are two common properties on all the Shape classes?Ans.There are more than a dozen properties on the base class System.Windows.Shapes.Shape.
Fill
Stroke
StrokeDashArray
StrokeDashCap
StrokeEndLineCap
StrokeLineJoin
StrokeMiterLimit
StrokeStartLineCap
StrokeThicknessQ.What are the different types of brushes that WPF offers?Ans.There are six different types of brushes
SolidColorBrush
LinearGradientBrush
RadialGradientBrush
DrawingBrush
VisualBrush
ImageBrushQ.What are the four channels of a WPF Color object?Ans.There are four channels of a WPF color objects are
Alpha
Red
Green
BlueQ.What are the types of bitmap effects?Ans.The built-in bitmap effects are
DropShadowBitmapEffect
OuterGlowBitmapEffect
BlurBitmapEffect
EmbossBitmapEffect
BevelBitmapEffectQ.What are the two types of transforms present on FrameworkElement?Ans.The two transforms that are present on FrameworkElement are RenderTransform and LayoutTransform.Q.What is Windows Workflow Foundation?Ans.WWF is a programming model for building workflow-enabled applications on windows. System.Workflow namespace has all the necessary modules to develop any type of workflow.Q.What is a Workflow?Ans.A Workflow is a set of activities, which is stored as model and they depict a process.Workflow is run by the Workflow runtime engine.
Triggers in Styles
Triggers define a list of setters that are executed if the specified condition is fulfilled. WPF knows three diferent types of triggers
- Property Triggers get active, when a property gets a specified value.
- Event Triggers get active, when a specified event is fired.
- Data Triggers get active, when a binding expression reaches a specified value.
Property Triggers
A property trigger activates a list of setters when a property gets a specified value. If the value changes the trigger undoes the setters.
<Style x:Key="styleWithTrigger" TargetType="Rectangle"> <Setter Property="Fill" Value="LightGreen" /> <Style.Triggers> <Trigger Property="IsMouseOver" Value="True"> <Setter Property="Fill" Value="Red" /> </Trigger> </Style.Triggers> </Style> <Rectangle Style="{StaticResource styleWithTrigger}"></Rectangle>
Event Triggers
Data Triggers
Multi Triggers
Trick to use triggers everywhere
Triggers are only available in styles and templates. Even if every control has a propertyTriggers
, it only works withEventTriggers
. If you want to use normal triggers outside a style or a template, you can use a little trick. Just wrap your code with the following snippet:
<Control> <Control.Template> <ControlTemplate> <!-- Triggers are available here --> </ControlTemplate> </Control.Template> </Control>
Commonly Asked WPF Interview Questions and Answers (Part 1)
In this aritcle on "Commonly Asked WPF Interview Questions and Answers (Part 1)", I will focus on very simple and basic WPF interview questions which a WPF developer should know. These WPF interview questions are based on general introduction of WPF, XAML, MVVM Pattern, Comparison of WPF and Silverlight, Layout Panels in WPF and WPF UserControls / CustomControls.
1. What is WPF?
The Windows Presentation Foundation is Microsofts next generation UI framework to create applications with a rich user experience. It is part of the .NET framework 3.0 and higher.
WPF combines application UIs, 2D graphics, 3D graphics, documents and multimedia into one single framework. Its vector based rendering engine uses hardware acceleration of modern graphic cards. This makes the UI faster, scalable and resolution independent.
2. What is XAML?
Extensible Application Markup Language, or XAML (pronounced "zammel"), is an XML-based markup language developed by Microsoft. XAML is the language behind the visual presentation of an application that you develop in Microsoft Expression Blend, just as HTML is the language behind the visual presentation of a Web page. Creating an application in Expression Blend means writing XAML code, either by hand or visually by working in the Design view of Expression Blend.
3. What is MVVM Pattren?
Model-View-ViewModel (MVVM) pattern splits the User Interface code into 3 conceptual parts - Model, View and ViewModel.
Model is a set of classes representing the data coming from the services or the database.
View is the code corresponding to the visual representation of the data the way it is seen and interacted with by the user.
ViewModel serves as the glue between the View and the Model. It wraps the data from the Model and makes it friendly for being presented and modified by the view. ViewModel also controls the View's interactions with the rest of the application
4. What is the difference between MVVM and MVC patterns?
MVVM Model-View ViewModel is similar to MVC, Model-View Controller.
The controller is replaced with a View Model. The View Model sits below the UI layer. The View Model exposes the data and command objects that the view needs. You could think of this as a container object that view goes to to get its data and actions from. The View Model pulls its data from the model.
5. What are the similarities and differences between WPF and Silverlight?
Similarities between Silverlight and WPF
1. Silverlight and WPF both use XAML.
2. Silverlight and WPF share same syntax, code and libraries.
Difference between WPF and Silverlight
1. WPF is tool for writing full-featured desktop applications that run on Windows while Silverlight is for web application.
2. WPF competes with Windows Forms only for writing desktop applications while Silverlight competes with Flash, Java web apps etc.
6. What is the need of layout panels in WPF?
Layout of controls is critical to an applications usability. Arranging controls based on fixed pixel coordinates may work for an limited enviroment, but as soon as you want to use it on different screen resolutions or with different font sizes it will fail. WPF provides a rich set built-in layout panels that help you to avoid the common pitfalls.
7. What are the different types of layout panels in WPF?
There are mainly 5 types of layout panels in WPF. These are:
Grid Panel
Stack Panel
Dock Panel
Wrap Panel
Canvas Panel
8. How to create a User Control and then how to use it in a WPF application?
User Controls are commonly used in a WPF application. So you should know this concept and you should be able to write a simple program to show how usercontrols are created and used in WPF application. I am sharing a link which created and uses usercontrol in very simple manner.
9. What is the difference between UserControl and CustomControl in WPF?
UserControl (Composition)
Composes multiple existing controls into a reusable "group"
Consists of a XAML and a code behind file
Cannot be styled/templated
Derives from UserControl
CustomControl (Extending an existing control)
Consists of a code file and a default style in Themes/Generic.xaml
Can be styled/templated
The best approach to build a control library
Nice post! I really enjoy this blog
ReplyDeleteDot Net Online Course Hyderabad