An extension method is a new language feature of C# starting with the 3.0 specification, as well as Visual Basic.NET starting with 9.0 and Oxygene with 2.0. Extension methods enable you to "add" methods to existing types without creating a new derived type, recompiling, or otherwise modifying the original type. Extension methods are a special kind of static method, but they are called as if they were instance methods on the extended type. For client code written in C# and Visual Basic, there is no apparent difference between calling an extension method and the methods that are actually defined in a type.
What are extension methods?
Extension methods enable you to add methods to existing types without creating a new derived type, recompiling, or otherwise modifying the original type.
An extension method is a special kind of static method, but they are called as if they were instance methods on the extended type.
How to use extension methods?
An extension method is a static method of a static class, where the "this" modifier is applied to the first parameter. The type of the first parameter will be the type that is extended.
Extension methods are only in scope when you explicitly import the namespace into your source code with a using directive.
Benefits of extension methods
- Extension methods allow existing classes to be extended without relying on inheritance or having to change the class's source code.
- If the class is sealed than there in no concept of extending its functionality. For this a new concept is introduced, in other words extension methods.
- This feature is important for all developers, especially if you would like to use the dynamism of the C# enhancements in your class's design.
Extension Methods Usage
This section is optional reading section for understanding the core idea of extension methods:
- This keyword has to be the first parameter in the extension method parameter list.
- Extension methods are used extensively in C# 3.0 and further version specially for LINQ extensions in C#, for example:
int[] ints = { 10, 45, 15, 39, 21, 26 }; var result = ints.OrderBy(g => g);
Here, order by is a sample for extension method from an instance ofIEnumerable<T>
interface, the expression inside the parenthesis is a lambda expression. - It is important to note that extension methods can't access the
private
methods in the extended type. - If you want to add new methods to a type, you don't have the source code for it, the ideal solution is to use and implement extension methods of that type.
- If you create extension methods that have the same signature methods inside the type you are extending, then the extension methods will never be called.
Important points for the use of extension methods
- An extension method must be defined in a top-level static class.
- An extension method with the same name and signature as an instance method will not be called.
- Extension methods cannot be used to override existing methods.
- The concept of extension methods cannot be applied to fields, properties or events.
- Overuse of extension methods is not a good style of programming.
Program to show how to use extension methods
Create a project Class Library as:
using System;
using System.Text;namespace ClassLibExtMethod
{
public class Class1 {
public string Display()
{
return ("I m in Display");
}
public string Print()
{
return ("I m in Print");
}
}
}
Now create a new project using "File" -> "New" -> "Project...".
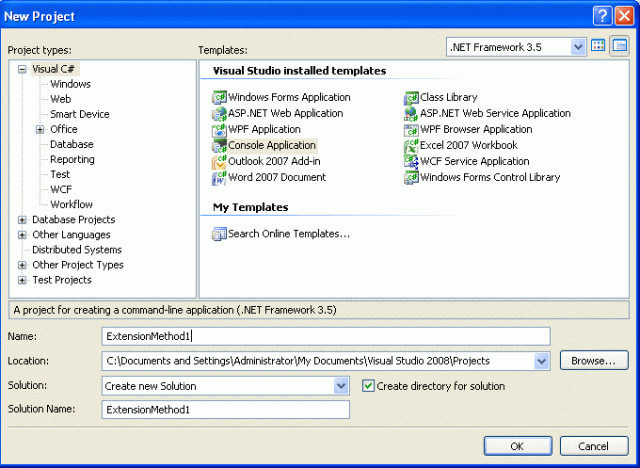
Add the reference of the previously created class library to this project.
Use the following code and use the ClassLibExtMEthod.dll in your namespace:using System;
using System.Text;
using ClassLibExtMethod;
namespace ExtensionMethod1
{
public static class XX
{
public static void NewMethod(this Class1 ob)
{
Console.WriteLine("Hello I m extended method");
}
}
class Program
{
static void Main(string[] args)
{
Class1 ob = new Class1();
ob.Display();
ob.Print();
ob.NewMethod();
Console.ReadKey();
}
}
}
You can see the extension method with an arrow (different from the normal method sign), since they were instance methods on the extended type. See the figure below:
Output of the preceding program
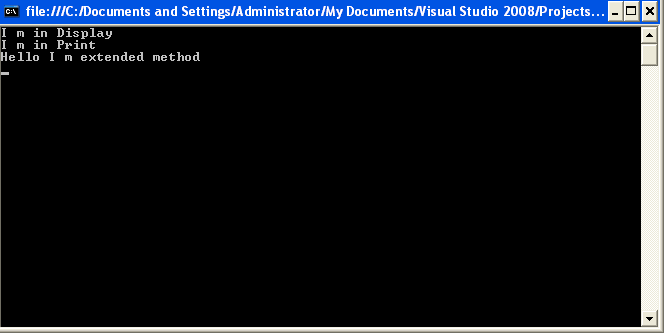
Benefits of extension methods
Create a project Class Library as:
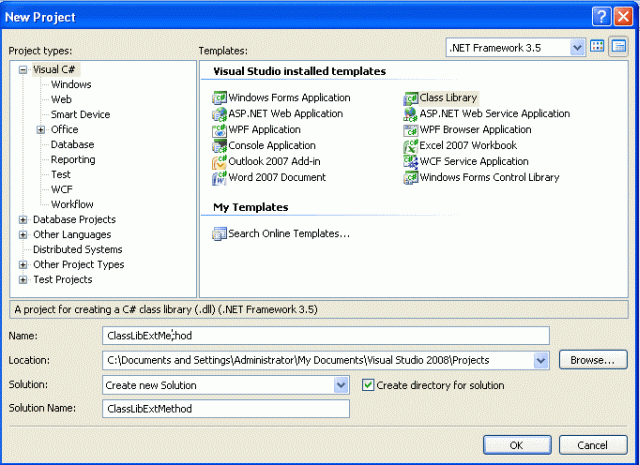
using System.Text;namespace ClassLibExtMethod
{
public class Class1 {
public string Display()
{
return ("I m in Display");
}
public string Print()
{
return ("I m in Print");
}
}
}
Now create a new project using "File" -> "New" -> "Project...".
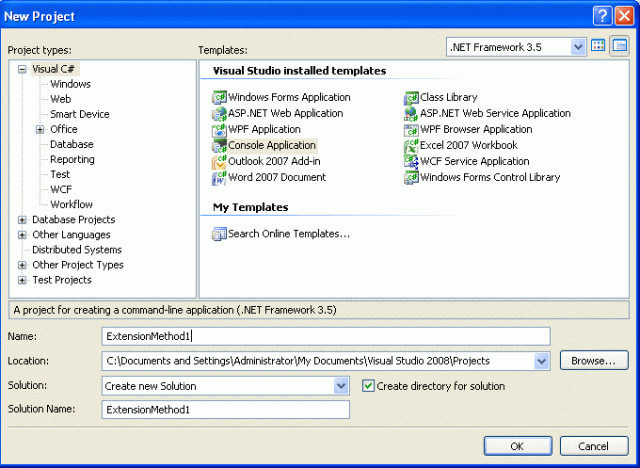
Add the reference of the previously created class library to this project.
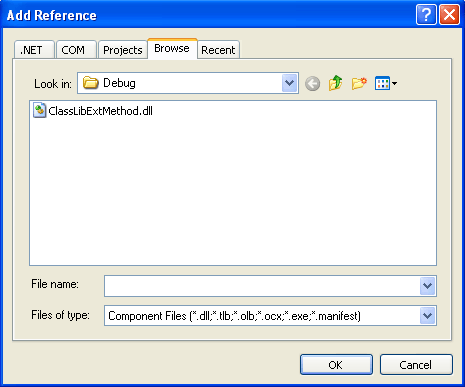
using System.Text;
using ClassLibExtMethod;
namespace ExtensionMethod1
{
public static class XX
{
public static void NewMethod(this Class1 ob)
{
Console.WriteLine("Hello I m extended method");
}
}
class Program
{
static void Main(string[] args)
{
Class1 ob = new Class1();
ob.Display();
ob.Print();
ob.NewMethod();
Console.ReadKey();
}
}
}
You can see the extension method with an arrow (different from the normal method sign), since they were instance methods on the extended type. See the figure below:
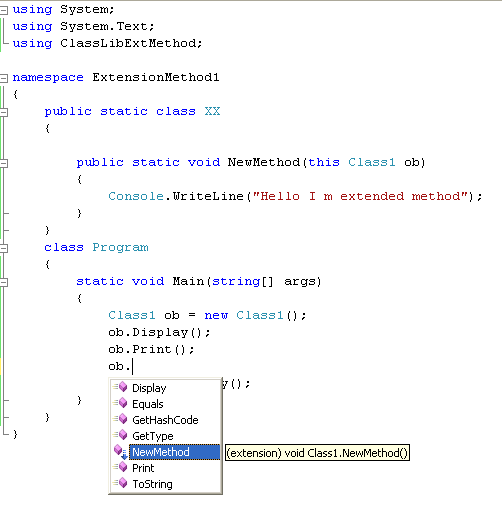
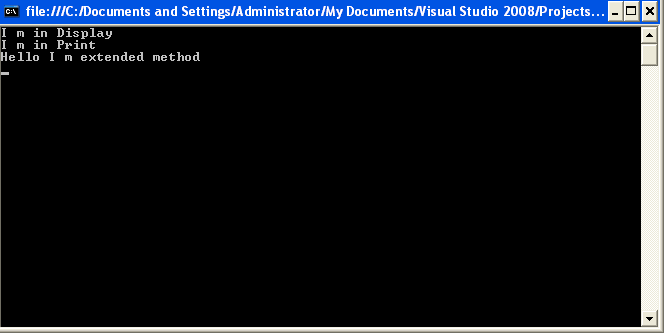
Benefits of extension methods
- Extension methods allow existing classes to be extended without relying on inheritance or having to change the class's source code.
- If the class is sealed than there in no concept of extending its functionality. For this a new concept is introduced, in other words extension methods.
- This feature is important for all developers, especially if you would like to use the dynamism of the C# enhancements in your class's design.
using System;
using System.Text;
namespace ExtensionMethod2
{
public static class ExtMetClass
{
public static int IntegerExtension(this string str)
{
return Int32.Parse(str);
}
}
class Program
{
static void Main(string[] args)
{
string str = "123456";
int num = str.IntegerExtension();
Console.WriteLine("The output using extension method: {0}", num);
Console.ReadLine();
}
}
}
Dot Net is the best programming language ever. Your blog have explained the excellent concept which helps me to gain more info on C# programming language.
ReplyDeleteRegards:
.net course in chennai
.net coaching centers in chennai
I simply wanted to thank you so much again. I am not sure the things that I might have gone through without the type of hints revealed by you regarding that situation.
ReplyDeleteHadoop Training Institute In chennai
That was a great message in my carrier, and It's wonderful commands like mind relaxes with understand words of knowledge by information's.
ReplyDeletejava training in chennai | java training in bangalore
java online training | java training in pune
java training in chennai | java training in bangalore
java training in tambaram |
Thanks for the good words! Really appreciated. Great post. I’ve been commenting a lot on a few blogs recently, but I hadn’t thought about my approach until you brought it up.
ReplyDeleteBlueprism training in Chennai
Blueprism training in Bangalore
Blueprism training in Pune
Blueprism training in tambaram
Blueprism training in annanagar
Blueprism training in velachery
Blueprism training in marathahalli
All the points you described so beautiful. Every time i read your i blog and i am so surprised that how you can write so well.
ReplyDeleteData Science Training in Chennai
Data science training in bangalore
Data science online training
Data science training in pune
Data science training in kalyan nagar
selenium training in chennai
Good Post! Thank you so much for sharing this pretty post, it was so good to read and useful to improve my knowledge as updated one, keep blogging.
ReplyDeleterpa training in Chennai
rpa training in anna nagar | rpa training in marathahalli
rpa training in btm | rpa training in kalyan nagar
rpa training in electronic city | rpa training in chennai
rpa online training | selenium training in training
I likable the posts and offbeat format you've got here! I’d wish many thanks for sharing your expertise and also the time it took to post!!
ReplyDeletepython online training
python training in OMR
python training in tambaram
python training in annanagar
This is most informative and also this post most user friendly and super navigation to all posts... Thank you so much for giving this information to me..
ReplyDeletejava course in chennai | java course in bangalore
java online training | java course in pune
This is a terrific article, and that I would really like additional info if you have got any. I’m fascinated with this subject and your post has been one among the simplest I actually have read.
ReplyDeleteangularjs Training in chennai
angularjs Training in chennai
angularjs-Training in tambaram
angularjs-Training in sholinganallur
angularjs-Training in velachery
The site was so nice, I found out about a lot of great things. I like the way you make your blog posts. Keep up the good work and may you gain success in the long run.
ReplyDeleteData Science training in Chennai
Data science training in bangalore
Data science training in pune
Data science online training
Good Post! Thank you so much for sharing this pretty post, it was so good to read and useful to improve my knowledge as updated one, keep blogging.
ReplyDeleteJava Training Classes
MSBI Training Classes
The site was so nice, I found out about a lot of great things. I like the way you make your blog posts. Keep up the good work and may you gain success in the long run.
ReplyDeleteScom2012 Training From India
Office365 Training From India
Great and nice blog thanks sharing..I just want to say that all the information you have given here is awesome...Thank you very much for this one.
ReplyDeleteApp-v Online Training
AWS Online Training
Azure Online Training
Business Analysis Online Training
Please let me know if you’re looking for an author for your site. You have some great posts, and I think I would be a good asset.
ReplyDeletesafety course in chennai
Informative post, thanks for sharing.
ReplyDeleteAngularjs courses in Chennai
Angular Training in Chennai
Angularjs Training in Chennai
Angular 6 Training in Chennai
RPA courses in Chennai
DevOps Training in Chennai
Nice post. Thanks for sharing such a worthy information.
ReplyDeleteSpoken English Classes in Chennai Saidapet
Spoken English Classes in KK Nagar
Spoken English Classes in Mylapore Chennai
Spoken English Coaching near me
Spoken English Class in Anna Nagar
Spoken English Classes in Arumbakkam
Spoken English Classes in Korattur
you have brainstormed my mind with your excellent blog. Thanks for that !
ReplyDeleteSelenium Training in Chennai
software testing selenium training
ios developer course in chennai
Digital Marketing Course in Chennai
Selenium Interview Questions and Answers
Future of testing professional
Cloud Courses in Chennai
Cloud Computing Classes in Chennai
Excellent Blog!!! Such an interesting blog with clear vision, this will definitely help for beginner to make them update.
ReplyDeletedata analytics training in bangalore
Data Science Courses in Bangalore
Best Data Science Courses in Bangalore
data analytics courses in bangalore
big data analytics training in bangalore
I really thank you for your innovative post.I have never read a creative ideas like your posts.here after i will follow your posts which is very much help for my career.
ReplyDeleteAWS Certification Training in Bangalore
AWS Certification Training
AWS Certification Training in Anna nagar
AWS Training in Guindy
ReplyDeleteThanks for sharing this pretty post, it was good and helpful.
Machine Learning Course in Chennai
Machine Learning course near me
Data Science Course in Chennai
Data Science Training in Chennai
Blue Prism Training in Chennai
UiPath Training in Chennai
ccna course in Chennai
R Programming Training in Chennai
ReplyDeleteYour post is great. It shows your deep understanding of the subject. Waiting for your future posts.
Pega training in chennai
Pega course in chennai
Pega training institutes in chennai
Pega course
Pega training
SAS Training in Chennai
SAS Course in Chennai
IELTS Coaching in Chennai
IELTS Training in Chennai
The knowledge of technology you have been sharing thorough this post is very much helpful to develop new idea. here by i also want to share this.
ReplyDeleteangularjs online training
apache spark online training
informatica mdm online training
devops online training
aws online training
Contact Your Best QuickBooks Enterprise Companion At QQuickBooks Enterprise Technical Support Number We all know that for the annoying issues in QuickBooks Enterprise software, you'll need an intelligent companion who are able to enable you to get rid of the errors instantly
ReplyDeleteQuickBooks Support we now have a tendency to rank our customers over something and that we aim to supply you a swish accounting and management expertise. you’ll additionally visit our web site to induce to grasp additional concerning our code and its upgrades.
ReplyDeleteIt does'nt matter if you're getting performance errors or you are facing any kind of trouble to upgrade your software to its latest version, you are able to quickly get help with QuickBooks Customer Service.
ReplyDeleteIn that case, Quickbooks online payroll support number provides 24/7 make it possible to our customer. Only you must do is make an individual call at our toll-free QuickBooks Desktop Payroll Support Phone Number . You could get resolve all of the major issues include installations problem, data access issue, printing related issue, software setup, server not responding error etc with our QuickBooks payroll support team.
ReplyDeleteQuickBooks Support Phone Number, there is absolutely no point in wasting your time and effort, getting worried for the problem you are facing an such like. Just call and you'll get instant respite from the difficulty due to various QuickBooks errors.
ReplyDeleteNow if you should be thinking what exactly is so new when you look at the 2019 pro, premier & enterprise versions that may enhance the payroll functionalities? AccountWizy may be the right location to keep yourself up-to-date regarding top accounting software. Dial our QuickBooks Enterprise Support Number to directly speak with our QuickBooks Experts to have tech support on Intuit Online & Full Service Payroll.
ReplyDeleteSignificant amount of features through the end are there any to guide both you and contribute towards enhancing your web business. Let’s see what QuickBooks Enterprise Support Number is approximately.
ReplyDeleteQuickBooks Customer Care Telephone Number: Readily Available For every QuickBooks Version
ReplyDeleteConsist of a beautiful bunch of accounting versions, viz., QuickBooks Pro, QuickBooks Premier, QuickBooks Enterprise, QuickBooks POS, QuickBooks Mac, QuickBooks Windows, and QuickBooks Payroll, QuickBooks has grown to become a dependable accounting software that one may tailor depending on your industry prerequisite. As well as it, our QuickBooks Support Phone Number
will bring in dedicated and diligent back-end helps for you for in case you find any inconveniences in operating any of these versions.
Our Professionals have designed services in a competent means in order that they will offer you the required ways to the shoppers. we now have a tendency to at QuickBooks Technical Support client Service are accessible 24*7 you just need to call our QuickBooks Support toll-free number which can be found in the marketplace on our website. Unneeded to mention, QuickBooks has given its utmost support to entrepreneurs in decreasing the purchase price otherwise we’ve seen earlier, however, an accountant wont to help keep completely different accounting record files. Utilizing the assistance of QuickBooks, users will maintain records like examining, recording and reviewing the complicated accounting procedures.
ReplyDeleteSignificant amount of features through the end are there any to guide both you and contribute towards enhancing your web business. Let’s see what QuickBooks Enterprise Tech Support Phone Number is approximately.
ReplyDeleteEven although you are feeling that the full time is odd to call for help, just pick up your phone and dial us at Support For QuickBooks because we offer our support services 24*7. We believe that the show must go on and thus time will not be a concern for all of us because problems do not come with any pre-announcements.
ReplyDeleteQuickBooks Technical Support Phone Number is assisted by our customer support representatives who answer your call instantly and resolve all your valuable issues at that moment.
ReplyDeleteQuickBooks Technical Support Number are often applied to one laptop or multiple computers. It is applied to the Windows software systems still as waterproof operative system platforms.
ReplyDeleteWe ensure that your calls aren't getting bounced. In case your calls are neglecting to relate with us at QuickBooks Support Phone Number Accounting Support Services, then you can certainly also join our team by dropping a message at our website without feeling shy.
ReplyDeleteWe urge you to definitely not ever suffer with losses due to longer downtime of your respective QB Premier. Simply get in touch with us at our website . and today we'll pro-actively resolve every one of the errors and issues faced by you in your type of QuickBooks Tech Support Phone Number Premier.
ReplyDeleteYou can further read the contact information on how to Contact Quickbooks Tech Support Phone Number . QuickBooks technical support team are active for only 5 days (Mon-Fri) in a week. The QuickBooks Premier Support Number cell phone number is obtainable these days from 6 AM to 6 PM.
ReplyDeleteQuickBooks made it easy for us to organize and manage all our any type of transactions and analyze profits. And if you are facing issues in the functioning of QuickBooks then easily get instant support from our QuicKbooks Customer Support Phone Number and highly qualified expert team.
ReplyDeleteQuickBooks Enterprise by Intuit offers extended properties and functionalities to users. It is specially developed in terms of wholesale, contract, nonprofit retail, and related industries. QuickBooks Enterprise Tech Support Number is preferred for users to offer you intuitive accounting means to fix SMEs running enterprise form of QuickBooks.
ReplyDeleteIt signifies that one can access our tech support for QuickBooks at any moment. Our backing team is dedicated enough to bestow you with end-to-end QuickBooks Support solutions when you desire to procure them for every single QuickBooks query.
ReplyDeleteWhen you get annoying errors in your QuickBooks Payroll Technical Support Number application and you’re not able to correct them, don’t lose yourself. Any QuickBooks errors can be fixed at our toll-free helpline through our Intuit’s QuickBooks ProAdvisors.
ReplyDeleteAre you thinking that how will you place your company finance in an organized way? Your research is completed now. QuickBooks Online Support is the right choice for you. Learn more about how To Create A Journal Entry In QuickBooks Support Phone Number Online?
ReplyDeleteyou must additionally get guidance and support services for the code that square measure obtainable 24/7. If just in case you come across any QuickBooks errors or problems or would like any facilitate, you’ll dial the direct line variety to achieve the QuickBooks Tech Support Phone Number specialists.
ReplyDeleteAnd also thanks for sharing this informative. Keep it Sir and I am waiting for your next post on your site blog.
ReplyDeleteBest Training Institute in Bangalore BTM. My Class Training Bangalore training center for certified course, learning on Software Training Course by expert faculties, also provides job placement for fresher, experience job seekers.
Software Training Institute in Bangalore
QuickBooks Enterprise is a high-functioning software designed specifically towards large organizations. It has multiple advanced features to help out accounting and bookkeeping professionals. If you would like to learn how to Troubleshoot Quickbooks Error 9999, you can continue reading this blog.
ReplyDeleteNice Blog. the Blog is really very Informative. every content should be neat and uniquely represented.
ReplyDeleteData Science Training Course In Chennai | Data Science Training Course In Anna Nagar | Data Science Training Course In OMR | Data Science Training Course In Porur | Data Science Training Course In Tambaram | Data Science Training Course In Velachery
Thanks for your blog. Really informative post. I hope i will find more interesting blog from you. I also like to share something interesting good luck.
ReplyDeleteAi & Artificial Intelligence Course in Chennai
PHP Training in Chennai
Ethical Hacking Course in Chennai Blue Prism Training in Chennai
UiPath Training in Chennai
"Thanks for sharing the wonderful post!
ReplyDeleteDigital Marketing Training Course in Chennai | Digital Marketing Training Course in Anna Nagar | Digital Marketing Training Course in OMR | Digital Marketing Training Course in Porur | Digital Marketing Training Course in Tambaram | Digital Marketing Training Course in Velachery
"
Great post i must say and thanks for the information. Education is definitely a sticky subject.
ReplyDeleteDigital Marketing Training Course in Chennai | Digital Marketing Training Course in Anna Nagar | Digital Marketing Training Course in OMR | Digital Marketing Training Course in Porur | Digital Marketing Training Course in Tambaram | Digital Marketing Training Course in Velachery
Dotnet Interview question and answer very useful through this website..Thanks for your good sharing!!
ReplyDeleteAndroid Training in Chennai | Certification | Mobile App Development Training Online | Android Training in Bangalore | Certification | Mobile App Development Training Online | Android Training in Hyderabad | Certification | Mobile App Development Training Online | Android Training in Coimbatore | Certification | Mobile App Development Training Online | Android Training in Online | Certification | Mobile App Development Training Online
Really nice article reader loved it Thank you.
ReplyDeleteTopic wise interview Question with answer
how an interviewer can ask one by one to know how deep knowledge you have.
visit Dot Net Interview Question Answer
This comment has been removed by the author.
ReplyDeletehere is the link for more Dot Net Interview Question And Answer
ReplyDeletedotnetmedium.blogspot.com
The Blog is really very Impressive.every content should be very neatly represented.Nice Blog ! It was really a nice article and i was really impressed by reading this. Thanks for sharing such detailed information.
ReplyDeleteData Science Training In Chennai
Data Science Online Training In Chennai
Data Science Training In Bangalore
Data Science Training In Hyderabad
Data Science Training In Coimbatore
Data Science Training
Data Science Online Training
Appreciation for really being thoughtful and also for deciding on certain marvelous guides most people really want to be aware of.
ReplyDeleteCyber Security Training Course in Chennai | Certification | Cyber Security Online Training Course | Ethical Hacking Training Course in Chennai | Certification | Ethical Hacking Online Training Course |
CCNA Training Course in Chennai | Certification | CCNA Online Training Course | RPA Robotic Process Automation Training Course in Chennai | Certification | RPA Training Course Chennai | SEO Training in Chennai | Certification | SEO Online Training Course
Sharing the same interest, Infycle feels so happy to share our detailed information about all these courses with you all! Do check them out
ReplyDeleteBig data training in chennai & get to know everything you want to about software trainings
ReplyDeleteTitle:
No.1 AWS Training Center in Chennai | Infycle Technologies
Description:
Learn Amazon Web Services for making your career as a shining sun with Infycle Technologies. Infycle Technologies is the best AWS training center in Chennai, providing complete hands-on practical training of professional specialists in the field. In addition to that, it also offers numerous programming language tutors in the software industry such as Oracle, Java, Python, AWS, Hadoop, etc. Once after the training, interviews will be arranged for the candidates, so that, they can set their career without any struggle. Of all that, 200% placement assurance will be given here. To have the best career, call 7502633633 to Infycle Technologies and grab a free demo to know more.
Best training in Chennai
perde modelleri
ReplyDeletesms onay
mobil ödeme bozdurma
NFT NASIL ALİNİR
Ankara Evden Eve Nakliyat
trafik sigortası
Dedektor
web sitesi kurma
aşk kitapları
pendik vestel klima servisi
ReplyDeleteataşehir arçelik klima servisi
maltepe samsung klima servisi
kadıköy samsung klima servisi
üsküdar samsung klima servisi
pendik bosch klima servisi
beykoz alarko carrier klima servisi
beykoz daikin klima servisi
üsküdar daikin klima servisi
Good content. You write beautiful things.
ReplyDeletehacklink
taksi
vbet
vbet
sportsbet
korsan taksi
sportsbet
mrbahis
mrbahis
Good text Write good content success. Thank you
ReplyDeletepoker siteleri
betmatik
tipobet
bonus veren siteler
kralbet
betpark
mobil ödeme bahis
kibris bahis siteleri
betmatik
ReplyDeletekralbet
betpark
mobil ödeme bahis
tipobet
slot siteleri
kibris bahis siteleri
poker siteleri
bonus veren siteler
70WQ6
https://saglamproxy.com
ReplyDeletemetin2 proxy
proxy satın al
knight online proxy
mobil proxy satın al
J6DZM
شركة مكافحة الحمام بالدمام NxxJ01FDYl
ReplyDeleteشركة مكافحة حشرات بالجبيل FQAqHEV9q4
ReplyDeleteشركة تنظيف مكيفات بحفر الباطن
ReplyDeleterObF8QJi3Y
مكافحة حشرات الاحساء
ReplyDeleteE9KjH0lFMw
صيانة افران الغاز بابها
ReplyDeleteYqiMEby7ya
Great post!
ReplyDeleteتنظيف افران الغاز مكة