Remoting
Establishing communication between objects that run in different processes, whether on the same computer or on computers thousands of miles apart, is a common development goal, especially when building widely-distributed applications. Traditionally, this has required in-depth knowledge not only of the objects on either end of the communication stream, but also of a host of lower-level protocols, application programming interfaces, and configuration tools or files. In short, it was a complex task demanding concentration and experience.
The .NET Framework makes several communication methods available to accomplish this task quickly and easily, even with minimal knowledge of protocols and encodings. As a result, whether you need to develop a Web application quickly or spend more time building a critical enterprise-wide application that involves many computers or operating systems and uses multiple protocols and serialization optimizations, the .NET Framework supports your scenario. Communicating across processes is still a complex task, but much of it is now handled by the .NET Framework.
.NET remoting enables client applications to use objects in other processes on the same computer or on any other computer available on its network. You can also use .NET remoting to communicate with other application domains in the same process. .NET remoting provides an abstract approach to interprocess communication that separates the remotable object from a specific server and client process and from a specific mechanism of communication. As a result, it is flexible and easily customizable. You can replace one communication protocol with another communication protocol, or one serialization format with another without recompiling the client or the server. In addition, the remoting system assumes no particular application model. You can communicate from a Web application, a console application, a Windows Service – from almost anything you want to use. Remoting servers can also be any type of executable application. Any application can host remoting objects and thus provide its services to any client on its computer or network
Distributed computing is an integral part of almost every software development. Before .Net Remoting, DCOM was the most used method of developing distributed application on Microsoft platform. Because of object oriented architecture, .NET Remoting replaces DCOM as .Net framework replaces COM.
Benefits of Distributed Application Development:
Fault Tolerance: Fault tolerance means that a system should be resilient when failures within the system occur.
Scalability: Scalability is the ability of a system to handle increased load with only an incremental change in performance.
Administration: Managing the system from one place.
In brief, .NET remoting is an architecture which enables communication between different application domains or processes using different transportation protocols, serialization formats, object lifetime schemes, and modes of object creation. Remote means any object which executes outside the application domain. The two processes can exist on the same computer or on two computers connected by a LAN or the Internet. This is called marshalling (This is the process of passing parameters from one context to another.), and there are two basic ways to marshal an object:
Marshal by value: the server creates a copy of the object passes the copy to the client.
Marshal by reference: the client creates a proxy for the object and then uses the proxy to access the object.
Comparison between .NET Remoting and Web services:
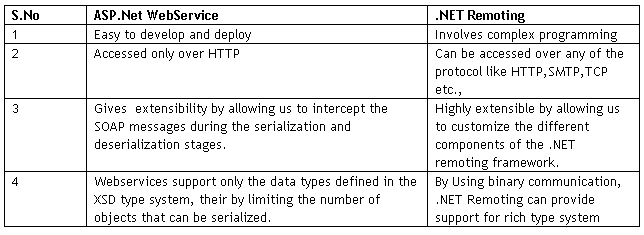
For performance comparison between .Net Remoting and ASP.Net Web Services Click.
Architecture:
Remote objects are accessed thro channels. Channels are Transport protocols for passing the messages between Remote objects. A channel is an object that makes communication between a client and a remote object, across app domain boundaries. The .NET Framework implements two default channel classes, as follows:
HttpChannel: Implements a channel that uses the HTTP protocol.
TcpChannel: Implements a channel that uses the TCP protocol (Transmission Control Protocol).
Channel take stream of data and creates package for a transport protocol and sends to other machine. A simple architecture of .NET remoting is as in Fig 1.
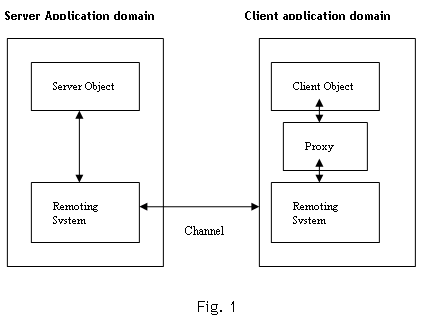
As Fig.1 shows, Remoting system creates a proxy for the server object and a reference to the proxy will be returned to the client. When client calls a method, Remoting system sends request thro the channel to the server. Then client receives the response sent by the server process thro the proxy.
Example:
Let us see a simple example which demonstrates .Net Remoting. In This example the Remoting object will send us the maximum of the two integer numbers sent.
Creating Remote Server and the Service classes on Machine 1:
Please note for Remoting support your service (Remote object) should be derived from MarshalByRefObject.
using System;
using System.Runtime.Remoting.Channels; //To support and handle Channel and channel sinksusing System.Runtime.Remoting;
using System.Runtime.Remoting.Channels.Http; //For HTTP channelusing System.IO;
namespace ServerApp
{
public class RemotingServer
{
public RemotingServer()
{
//// TODO: Add constructor logic here//}
}
//Service classpublic class Service: MarshalByRefObject
{
public void WriteMessage (int num1,int num2)
{
Console.WriteLine (Math.Max(num1,num2));
}
}
//Server Classpublic class Server
{
public static void Main ()
{
HttpChannel channel = new HttpChannel(8001); //Create a new channelChannelServices.RegisterChannel (channel); //Register channelRemotingConfiguration.RegisterWellKnownServiceType(typeof Service),"Service",WellKnownObjectMode.Singleton);
Console.WriteLine ("Server ON at port number:8001");
Console.WriteLine ("Please press enter to stop the server.");
Console.ReadLine ();
}
}
}
Save the above file as ServerApp.cs. Create an executable by using Visual Studio.Net command prompt by,
csc /r:system.runtime.remoting.dll /r:system.dll ServerApp.cs
A ServerApp.Exe will be generated in the Class folder.
Run the ServerApp.Exe will give below message on the console
Server ON at port number:8001
Please press enter to stop the server.
In order to check whether the HTTP channel is binded to the port, type http://localhost:8001/Service?WSDL in the browser.
You should see a XML file describing the Service class.
Please note before running above URL on the browser your server (ServerApp.Exe should be running) should be ON.
Creating Proxy and the Client application on Machine 2
SoapSuds.exe is a utility which can be used for creating a proxy dll.
Type below command on Visual studio.Net command prompt.
soapsuds -url:http://< Machine Name where service is running>:8001/Service?WSDL -oa:Server.dll
This will generates a proxy dll by name Server.dll. This will be used to access remote object.
Client Code:
using System;
using System.Runtime.Remoting.Channels; //To support and handle Channel and channel sinksusing System.Runtime.Remoting;
using System.Runtime.Remoting.Channels.Http; //For HTTP channelusing System.IO;
using ServerApp;
namespace RemotingApp
{
public class ClientApp
{
public ClientApp()
{
}
public static void Main (string[] args)
{
HttpChannel channel = new HttpChannel (8002); //Create a new channelChannelServices.RegisterChannel (channel); //Register the channel//Create Service class objectService svc = (Service) Activator.GetObject (typeof (Service),"http://<Machine name where Service running>:8001/Service"); //Localhost can be replaced by //Pass Messagesvc.WriteMessage (10,20);
}
}
}
Save the above file as ClientApp.cs. Create an executable by using Visual Studio.Net command prompt by,
csc /r:system.runtime.remoting.dll /r:system.dll ClientrApp.cs
A ClientApp.Exe will be generated in the Class folder. Run ClientApp.Exe , we can see the result on Running ServerApp.EXE command prompt.
Any object can be changed into a remote object by deriving it from
Marshall by value has several implications; first, the entire remote object is transmitted on the network. Second, some or the entire remote object may have no relevance outside of its local context. For example, the remote object may have a connection to a database, or a handle to a window, or a file handle etc. Third, parts of the remote object may not be serialiazable. In addition, when the client invokes a method on an MBV object, the local machine does the execution, which means that the compiled code (remote class) has to be available to the client. Because the object exists entirely in the caller's application domain, no state changes to the object are communicated to the originating application domain, or from the originator back to the caller.
MBV objects are, however, very efficient if they are small, and provide a repeated function that does not consume bandwidth. The entire object exists in the caller's domain, so there is no need to marshal accesses across domain boundaries. Using marshal-by-value objects can increase performance and reduce network traffic, when used for small objects or objects to which you will be making many accesses.
Marshal by value classes must either be marked with the
Marshal by reference classes must inherit from
Channel selection is subject to the following rules:
Formatters are used for encoding and decoding the messages before they are transported by the channel. There are two native formatters in the .NET runtime, namely binary (
A key point to remember is that .NET Remoting always uses binary serialization, but you have your choice of output formats. The type of serialization (binary or XML) determines what object data is output. The formatter determines the format in which that data is stored.
The data in binary format has smaller size then in XML format. The smaller size makes binary formatting the obvious choice for intranet applications or whenever network performance is critical. However, not all firewalls look kindly on binary formatted data, so if you're distributing an application that needs to use Remoting over the Internet, you might find yourself forced to use SOAP formatting. Another benefit of SOAP formatting is that it's human readable, which gives you the opportunity to examine message traffic for debugging. Fortunately, Remoting works exactly the same regardless of which formatter you use, so you can use SOAP formatting during initial development and debugging, and then convert to binary format for production release.
The client can obtain the metadata information required to access the remote object in the following ways:
When to use which kind of activation depends upon different scenarios. Some of them are:
Establishing communication between objects that run in different processes, whether on the same computer or on computers thousands of miles apart, is a common development goal, especially when building widely-distributed applications. Traditionally, this has required in-depth knowledge not only of the objects on either end of the communication stream, but also of a host of lower-level protocols, application programming interfaces, and configuration tools or files. In short, it was a complex task demanding concentration and experience.
The .NET Framework makes several communication methods available to accomplish this task quickly and easily, even with minimal knowledge of protocols and encodings. As a result, whether you need to develop a Web application quickly or spend more time building a critical enterprise-wide application that involves many computers or operating systems and uses multiple protocols and serialization optimizations, the .NET Framework supports your scenario. Communicating across processes is still a complex task, but much of it is now handled by the .NET Framework.
.NET remoting enables client applications to use objects in other processes on the same computer or on any other computer available on its network. You can also use .NET remoting to communicate with other application domains in the same process. .NET remoting provides an abstract approach to interprocess communication that separates the remotable object from a specific server and client process and from a specific mechanism of communication. As a result, it is flexible and easily customizable. You can replace one communication protocol with another communication protocol, or one serialization format with another without recompiling the client or the server. In addition, the remoting system assumes no particular application model. You can communicate from a Web application, a console application, a Windows Service – from almost anything you want to use. Remoting servers can also be any type of executable application. Any application can host remoting objects and thus provide its services to any client on its computer or network
Distributed computing is an integral part of almost every software development. Before .Net Remoting, DCOM was the most used method of developing distributed application on Microsoft platform. Because of object oriented architecture, .NET Remoting replaces DCOM as .Net framework replaces COM.
Benefits of Distributed Application Development:
Fault Tolerance: Fault tolerance means that a system should be resilient when failures within the system occur.
Scalability: Scalability is the ability of a system to handle increased load with only an incremental change in performance.
Administration: Managing the system from one place.
In brief, .NET remoting is an architecture which enables communication between different application domains or processes using different transportation protocols, serialization formats, object lifetime schemes, and modes of object creation. Remote means any object which executes outside the application domain. The two processes can exist on the same computer or on two computers connected by a LAN or the Internet. This is called marshalling (This is the process of passing parameters from one context to another.), and there are two basic ways to marshal an object:
Marshal by value: the server creates a copy of the object passes the copy to the client.
Marshal by reference: the client creates a proxy for the object and then uses the proxy to access the object.
Comparison between .NET Remoting and Web services:
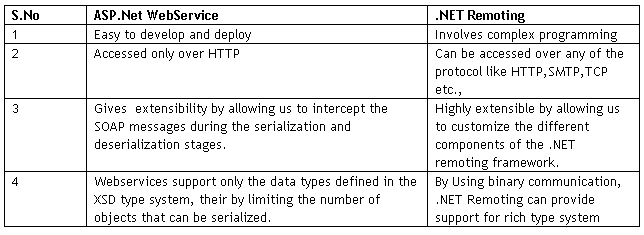
For performance comparison between .Net Remoting and ASP.Net Web Services Click.
Architecture:
Remote objects are accessed thro channels. Channels are Transport protocols for passing the messages between Remote objects. A channel is an object that makes communication between a client and a remote object, across app domain boundaries. The .NET Framework implements two default channel classes, as follows:
HttpChannel: Implements a channel that uses the HTTP protocol.
TcpChannel: Implements a channel that uses the TCP protocol (Transmission Control Protocol).
Channel take stream of data and creates package for a transport protocol and sends to other machine. A simple architecture of .NET remoting is as in Fig 1.
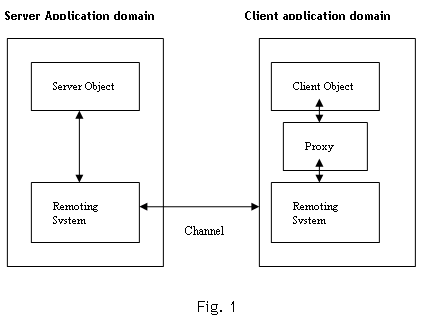
As Fig.1 shows, Remoting system creates a proxy for the server object and a reference to the proxy will be returned to the client. When client calls a method, Remoting system sends request thro the channel to the server. Then client receives the response sent by the server process thro the proxy.
Example:
Let us see a simple example which demonstrates .Net Remoting. In This example the Remoting object will send us the maximum of the two integer numbers sent.
Creating Remote Server and the Service classes on Machine 1:
Please note for Remoting support your service (Remote object) should be derived from MarshalByRefObject.
using System;
using System.Runtime.Remoting.Channels; //To support and handle Channel and channel sinksusing System.Runtime.Remoting;
using System.Runtime.Remoting.Channels.Http; //For HTTP channelusing System.IO;
namespace ServerApp
{
public class RemotingServer
{
public RemotingServer()
{
//// TODO: Add constructor logic here//}
}
//Service classpublic class Service: MarshalByRefObject
{
public void WriteMessage (int num1,int num2)
{
Console.WriteLine (Math.Max(num1,num2));
}
}
//Server Classpublic class Server
{
public static void Main ()
{
HttpChannel channel = new HttpChannel(8001); //Create a new channelChannelServices.RegisterChannel (channel); //Register channelRemotingConfiguration.RegisterWellKnownServiceType(typeof Service),"Service",WellKnownObjectMode.Singleton);
Console.WriteLine ("Server ON at port number:8001");
Console.WriteLine ("Please press enter to stop the server.");
Console.ReadLine ();
}
}
}
Save the above file as ServerApp.cs. Create an executable by using Visual Studio.Net command prompt by,
csc /r:system.runtime.remoting.dll /r:system.dll ServerApp.cs
A ServerApp.Exe will be generated in the Class folder.
Run the ServerApp.Exe will give below message on the console
Server ON at port number:8001
Please press enter to stop the server.
In order to check whether the HTTP channel is binded to the port, type http://localhost:8001/Service?WSDL in the browser.
You should see a XML file describing the Service class.
Please note before running above URL on the browser your server (ServerApp.Exe should be running) should be ON.
Creating Proxy and the Client application on Machine 2
SoapSuds.exe is a utility which can be used for creating a proxy dll.
Type below command on Visual studio.Net command prompt.
soapsuds -url:http://< Machine Name where service is running>:8001/Service?WSDL -oa:Server.dll
This will generates a proxy dll by name Server.dll. This will be used to access remote object.
Client Code:
using System;
using System.Runtime.Remoting.Channels; //To support and handle Channel and channel sinksusing System.Runtime.Remoting;
using System.Runtime.Remoting.Channels.Http; //For HTTP channelusing System.IO;
using ServerApp;
namespace RemotingApp
{
public class ClientApp
{
public ClientApp()
{
}
public static void Main (string[] args)
{
HttpChannel channel = new HttpChannel (8002); //Create a new channelChannelServices.RegisterChannel (channel); //Register the channel//Create Service class objectService svc = (Service) Activator.GetObject (typeof (Service),"http://<Machine name where Service running>:8001/Service"); //Localhost can be replaced by //Pass Messagesvc.WriteMessage (10,20);
}
}
}
Save the above file as ClientApp.cs. Create an executable by using Visual Studio.Net command prompt by,
csc /r:system.runtime.remoting.dll /r:system.dll ClientrApp.cs
A ClientApp.Exe will be generated in the Class folder. Run ClientApp.Exe , we can see the result on Running ServerApp.EXE command prompt.
Remote Objects
What are remote objects? Any object outside the application domain of
the caller application should be considered remote, where the object
will be reconstructed. Local objects that cannot be serialized cannot be
passed to a different application domain, and are therefore non
remotable.Any object can be changed into a remote object by deriving it from
MarshalByRefObject
, or by making it serializable either by adding the [Serializable]
tag or by implementing the ISerializable
interface. When a client activates a remote object, it receives a proxy
to the remote object. All operations on this proxy are appropriately
indirected to enable the Remoting infrastructure to intercept and
forward the calls appropriately. In cases where the proxy and remote
objects are in different application domains, all method call parameters
on the stack are converted into messages and transported to the remote
application domain, where the messages are turned back into a stack
frame and the method call is invoked. The same procedure is used for
returning results from the method call.
Types of .NET Remotable Objects
There are three types of objects that can be configured to serve as
.NET remote objects. You can choose the type of object depending on the
requirement of your application. Single Call
Single Call objects service one and only one request coming in. Single Call objects are useful in scenarios where the objects are required to do a finite amount of work. Single Call objects are usually not required to store state information, and they cannot hold state information between method calls.Singleton Objects
Singleton objects are those objects that service multiple clients, and hence share data by storing state information between client invocations. They are useful in cases in which data needs to be shared explicitly between clients, and also in which the overhead of creating and maintaining objects is substantial.Client-Activated Objects (CAO)
Client-activated objects (CAO) are server-side objects that are activated upon request from the client. When the client submits a request for a server object using a "new
"
operator, an activation request message is sent to the remote
application. The server then creates an instance of the requested class,
and returns an ObjRef
back to the client application that invoked it. A proxy is then created on the client side using the ObjRef
.
The client's method calls will be executed on the proxy.
Client-activated objects can store state information between method
calls for its specific client, and not across different client objects.
Each invocation of "new
" returns a proxy to an independent instance of the server type. Domains
In .NET, when an application is loaded in memory, a process is created, and within this process, an application domain is created. The application is actually loaded in the application domain. If this application communicates with another application, it has to use Remoting because the other application will have its own domain, and across domains, object cannot communicate directly. Different application domains may exist in same process, or they may exist in different processes.Contexts
The .NET runtime further divides the application domain into contexts. A context guarantees that a common set of constraints and usage semantics govern all access to the objects within it. All applications have a default context in which objects are constructed, unless otherwise instructed. A context, like an application domain, forms a .NET Remoting boundary. Access requests must be marshaled across contexts.Proxies
When a call is made between objects in the same Application Domain, only a normal local call is required; however, a call across Application Domains requires a remote call. In order to facilitate a remote call, a proxy is introduced by the .NET framework at the client side. This proxy is an instance of theTransparentProxy
class,
directly available to the client to communicate with the remote object.
Generally, a proxy object is an object that acts in place of some other
object. The proxy object ensures that all calls made on the proxy are
forwarded to the correct remote object instance. In .NET Remoting, the
proxy manages the marshaling process and the other tasks required to
make cross-boundary calls. The .NET Remoting infrastructure
automatically handles the creation and management of proxies.RealProxy and TransparentProxy
The .NET Remoting Framework uses two proxy objects to accomplish its work of making a remote call from a client object to a remote server object: aRealProxy
object and a TransparentProxy
object. The RealProxy
object does the work of actually sending messages to the remote object
and receiving response messages from the remote object. The TransparentProxy
interacts with the client, and does the work of intercepting the remote method call made by the client.Marshaling
Object Marshalling specifies how a remote object is exposed to the client application. It is the process of packaging an object access request in one application domain and passing that request to another domain. The .NET Remoting infrastructure manages the entire marshaling process. There are two methods by which a remote object can be made available to a local client object: Marshal by value, and Marshal by reference.Marshalling objects by value
Marshaling by value is analogous to having a copy of the server object at the client. Objects that are marshaled by value are created on the remote server, serialized into a stream, and transmitted to the client where an exact copy is reconstructed. Once copied to the caller's application domain (by the marshaling process), all method calls and property accesses are executed entirely within that domain.Marshall by value has several implications; first, the entire remote object is transmitted on the network. Second, some or the entire remote object may have no relevance outside of its local context. For example, the remote object may have a connection to a database, or a handle to a window, or a file handle etc. Third, parts of the remote object may not be serialiazable. In addition, when the client invokes a method on an MBV object, the local machine does the execution, which means that the compiled code (remote class) has to be available to the client. Because the object exists entirely in the caller's application domain, no state changes to the object are communicated to the originating application domain, or from the originator back to the caller.
MBV objects are, however, very efficient if they are small, and provide a repeated function that does not consume bandwidth. The entire object exists in the caller's domain, so there is no need to marshal accesses across domain boundaries. Using marshal-by-value objects can increase performance and reduce network traffic, when used for small objects or objects to which you will be making many accesses.
Marshal by value classes must either be marked with the
[Serilaizable]
attribute in order to use the default serialization, or must implement the ISerializable
interface.Marshalling objects by reference
Marshaling by reference is analogous to having a pointer to the object. Marshal by reference passes a reference to the remote object back to the client. This reference is anObjRef
class that
contains all the information required to generate the proxy object that
does the communication with the actual remote object. On the network,
only parameters and return values are passed. A remote method invocation
requires the remote object to call its method on the remote host
(server).Marshal by reference classes must inherit from
System.MarshalByRefObject
.Marshalling done by the .NET Framework
Marshalling is done by the .NET Framework itself. We don’t have to write any code for it.Channels
The .NET Remoting infrastructure provides a mechanism by which a stream of bytes is sent from one point to the other (client to server etc.). This is achieved via a channel. Strictly speaking, it is a class that implements theIChannel
interface. There are two pre-defined .NET Remoting channels existing in System.Runtime.Remoting.Channels
, the TcpChannel
and the HttpChannel
. To use the TcpChannel
, the server must instantiate and register the TcpServerChannel
class, and the client, the TcpClientChannel
class.Channel selection is subject to the following rules:
- At least one channel must be registered with the remoting framework before a remote object can be called. Channels must be registered before objects are registered.
- Channels are registered per application domain. There can be multiple application domains in a single process. When a process dies, all channels that it registers are automatically destroyed.
- It is illegal to register the same channel that listens on the same port more than once. Even though channels are registered per application domain, different application domains on the same machine cannot register the same channel listening on the same port. You can register the same channel listening on two different ports for an application domain.
- Clients can communicate with a remote object using any
registered channel. The remoting framework ensures that the remote
object is connected to the right channel when a client attempts to
connect to it. The client is responsible for calling the
RegisterChannel
on theChannelService
class before attempting to communicate with a remote object.
Serialization Formatters
.NET Remoting uses serialization to copy marshal-by-value objects, and to send reference of objects which are marshal-by-reference, between application domains. The .NET Framework supports two kinds of serialization: binary serialization and XML serialization.Formatters are used for encoding and decoding the messages before they are transported by the channel. There are two native formatters in the .NET runtime, namely binary (
System.Runtime.Serialization.Formatters.Binary
) and SOAP (System.Runtime.Serialization.Formatters.Soap
).
Applications can use binary encoding where performance is critical, or
XML encoding where interoperability with other remoting frameworks is
essential.A key point to remember is that .NET Remoting always uses binary serialization, but you have your choice of output formats. The type of serialization (binary or XML) determines what object data is output. The formatter determines the format in which that data is stored.
The data in binary format has smaller size then in XML format. The smaller size makes binary formatting the obvious choice for intranet applications or whenever network performance is critical. However, not all firewalls look kindly on binary formatted data, so if you're distributing an application that needs to use Remoting over the Internet, you might find yourself forced to use SOAP formatting. Another benefit of SOAP formatting is that it's human readable, which gives you the opportunity to examine message traffic for debugging. Fortunately, Remoting works exactly the same regardless of which formatter you use, so you can use SOAP formatting during initial development and debugging, and then convert to binary format for production release.
.NET Remoting Metadata
.NET Remoting uses metadata to dynamically create proxy objects. The proxy objects that are created at the client side have the same members as the original class. But the implementation of the proxy object just forwards all requests through the .NET Remoting runtime to the original object. The serialization formatter uses metadata to convert method calls to payload stream and back.The client can obtain the metadata information required to access the remote object in the following ways:
- The .NET assembly of the server object.
- The Remoting object can provide a WSDL (Web Services Description Language) file that describes the object and its methods.
- .NET clients can use the SOAPSUDS utility to download the XML schema from the server (generated on the server) to generate source files or an assembly that contains only metadata, no code.
Object Activation
Object activation refers to the various ways in which a remote object can be instantiated. Marshal by value objects have a simple activation scheme, they are created when the client first requests them. Marshal by reference objects have two activation schemes:Server Activated Objects
Server Activated Objects are created only when the client makes the first call to the remote method. In other words, when the client asks for the creation of the remote object, only the local proxy is created on the client, the actual remote object on the server is instantiated on the first method call. These proxies can be generated as:...
// On the Server
RemotingConfiguration.RegisterWellKnownServiceType(
typeof (RemoteServerObject), "Test",
WellKnownObjectMode.SingleCall);
...
// On the Client
IRemoteCom obj = (IRemoteCom)Activator.GetObject(typeof(IRemoteCom),
"tcp://localhost:1002/Test");
...
We have two registration types, Singleton
and SingleCall
, which were explained earlier. The code for both is:RemotingConfiguration.RegisterWellKnownServiceType( typeof(RemoteServerObject),
"Test", WellKnownObjectMode.SingleCall);
...
RemotingConfiguration.RegisterWellKnownServiceType( typeof(RemoteServerObject),
"Test", WellKnownObjectMode.Singleton);
For Server Activated Objects, the proxy object (on the client) and
the actual object (on the server) are created at different times.Client Activated Objects
These are created on the server immediately upon the client's request. An instance of a Client Activated Object is created every time the client instantiates one, either by the use ofnew
or Activator.CreateInstance(...)
....
// On the Server
RemotingConfiguration.ApplicationName = "TestCAO";
RemotingConfiguration.RegisterActivatedServiceType(typeof(RemoteObjectCAO));
...
// On the Client
RemotingConfiguration.RegisterActivatedClientType(typeof(RemoteObjectCAO),
"tcp://localhost:1002/TestCAO");
obj = new RemoteObjectCAO();
...
In all these examples, "localhost" can be replaced with an actual remote machine's IP address.When to use which kind of activation depends upon different scenarios. Some of them are:
- Singleton object reference to the same server object, and any changes made to that object by one client are visible by all of the object's clients. Think of the Windows Desktop, where a change made by one program is visible by all client programs. Persistent data is held on the server, and is accessible by all clients.
- Use the
SingleCall
model to provide a stateless programming model (the traditional Web services request/response model), or any time you have no need to maintain a persistent object state on the server. - Use client activation if the object needs to maintain persistent per-client state information.
No comments:
Post a Comment